diff --git a/README.md b/README.md
index 1c7c652d..683b56f0 100644
--- a/README.md
+++ b/README.md
@@ -9,12 +9,12 @@
[](https://discord.gg/zt2mTPcu)
-## ⚡⚡⚡ The PyTorch Library for Large language Model (LLM) Applications ⚡⚡⚡
+### ⚡ The PyTorch Library for Large language Model (LLM) Applications ⚡
*LightRAG* helps developers with both building and optimizing *Retriever-Agent-Generator (RAG)* pipelines.
It is *light*, *modular*, and *robust*.
-
+
+## LightRAG Task Pipeline
+
-**LightRAG**
+We will ask the model to respond with ``explaination`` and ``example`` of a concept. And we will build a pipeline to get the structured output as ``QAOutput``.
```python
-from lightrag.core import Component, Generator
-from lightrag.components.model_client import GroqAPIClient
+from dataclasses import dataclass, field
-class SimpleQA(Component):
- def __init__(self):
- super().__init__()
- template = r"""
- You are a helpful assistant.
-
- User: {{input_str}}
- You:
- """
- self.generator = Generator(
+from lightrag.core import Component, Generator, DataClass, fun_to_component, Sequential
+from lightrag.components.model_client import GroqAPIClient
+from lightrag.components.output_parsers import JsonOutputParser
+
+@dataclass
+class QAOutput(DataClass):
+ explaination: str = field(
+ metadata={"desc": "A brief explaination of the concept in one sentence."}
+ )
+ example: str = field(metadata={"desc": "An example of the concept in a sentence."})
+
+
+@fun_to_component
+def to_qa_output(data: dict) -> QAOutput:
+ return QAOutput.from_dict(data)
+
+
+class QA(Component):
+ def __init__(self):
+ super().__init__()
+ template = r"""
+You are a helpful assistant.
+
+{{output_format_str}}
+
+
+User: {{input_str}}
+You:
+ """
+ parser = JsonOutputParser(data_class=QAOutput)
+ self.generator = Generator(
model_client=GroqAPIClient(),
model_kwargs={"model": "llama3-8b-8192"},
template=template,
+ prompt_kwargs={"output_format_str": parser.format_instructions()},
+ output_processors=Sequential(parser, to_qa_output),
+ )
+
+ def call(self, query: str):
+ return self.generator.call({"input_str": query})
+
+ async def acall(self, query: str):
+ return await self.generator.acall({"input_str": query})
+```
+
+
+Run the following code for visualization and calling the model.
+
+```python
+
+qa = QA()
+print(qa)
+
+# call
+output = qa("What is LLM?")
+print(output)
+```
+
+**Structure of the pipeline**
+
+Here is what we get from ``print(qa)``:
+
+```
+QA(
+ (generator): Generator(
+ model_kwargs={'model': 'llama3-8b-8192'},
+ (prompt): Prompt(
+ template:
+ You are a helpful assistant.
+
+ {{output_format_str}}
+
+
+ User: {{input_str}}
+ You:
+ , prompt_kwargs: {'output_format_str': 'Your output should be formatted as a standard JSON instance with the following schema:\n```\n{\n "explaination": "A brief explaination of the concept in one sentence. (str) (required)",\n "example": "An example of the concept in a sentence. (str) (required)"\n}\n```\n-Make sure to always enclose the JSON output in triple backticks (```). Please do not add anything other than valid JSON output!\n-Use double quotes for the keys and string values.\n-Follow the JSON formatting conventions.'}, prompt_variables: ['output_format_str', 'input_str']
+ )
+ (model_client): GroqAPIClient()
+ (output_processors): Sequential(
+ (0): JsonOutputParser(
+ data_class=QAOutput, examples=None, exclude_fields=None
+ (json_output_format_prompt): Prompt(
+ template: Your output should be formatted as a standard JSON instance with the following schema:
+ ```
+ {{schema}}
+ ```
+ {% if example %}
+ Examples:
+ ```
+ {{example}}
+ ```
+ {% endif %}
+ -Make sure to always enclose the JSON output in triple backticks (```). Please do not add anything other than valid JSON output!
+ -Use double quotes for the keys and string values.
+ -Follow the JSON formatting conventions., prompt_variables: ['schema', 'example']
+ )
+ (output_processors): JsonParser()
)
+ (1): ToQaOutputComponent(fun_name=to_qa_output)
+ )
+ )
+)
+```
+
+**The output**
+
+Here is what we get from ``print(output)``:
+
+```
+GeneratorOutput(data=QAOutput(explaination='LLM stands for Large Language Model, which refers to a type of artificial intelligence designed to process and generate human-like language.', example='For example, a LLM can be trained to generate news articles, conversations, or even entire books, and can be used for a variety of applications such as language translation, text summarization, and chatbots.'), error=None, usage=None, raw_response='```\n{\n "explaination": "LLM stands for Large Language Model, which refers to a type of artificial intelligence designed to process and generate human-like language.",\n "example": "For example, a LLM can be trained to generate news articles, conversations, or even entire books, and can be used for a variety of applications such as language translation, text summarization, and chatbots."\n}', metadata=None)
+```
+**See the prompt**
+
+Use the following code:
+
+```python
+
+qa2.generator.print_prompt(
+ output_format_str=qa2.generator.output_processors[0].format_instructions(),
+ input_str="What is LLM?",
+)
+```
- def call(self, query):
- return self.generator({"input_str": query})
- async def acall(self, query):
- return await self.generator.acall({"input_str": query})
+The output will be:
+
+```
+You are a helpful assistant.
+
+Your output should be formatted as a standard JSON instance with the following schema:
```
+{
+ "explaination": "A brief explaination of the concept in one sentence. (str) (required)",
+ "example": "An example of the concept in a sentence. (str) (required)"
+}
+```
+-Make sure to always enclose the JSON output in triple backticks (```). Please do not add anything other than valid JSON output!
+-Use double quotes for the keys and string values.
+-Follow the JSON formatting conventions.
+
+
+User: What is LLM?
+You:
+```
+
## Quick Install
@@ -90,7 +215,7 @@ LightRAG full documentation available at [lightrag.sylph.ai](https://lightrag.sy
- [Introduction](https://lightrag.sylph.ai/)
- [Full installation guide](https://lightrag.sylph.ai/get_started/installation.html)
- [Design philosophy](https://lightrag.sylph.ai/developer_notes/lightrag_design_philosophy.html): Design based on three principles: Simplicity over complexity, Quality over quantity, and Optimizing over building.
-- [Class hierarchy](https://lightrag.sylph.ai/developer_notes/class_hierarchy.html): We have no more than two levels of subclasses. The bare minimum abstraction will developers with maximum customizability and simplicity.
+- [Class hierarchy](https://lightrag.sylph.ai/developer_notes/class_hierarchy.html): We have no more than two levels of subclasses. The bare minimum abstraction provides developers with maximum customizability and simplicity.
- [Tutorials](https://lightrag.sylph.ai/developer_notes/index.html): Learn the `why` and `how-to` (customize and integrate) behind each core part within the `LightRAG` library.
- [API reference](https://lightrag.sylph.ai/apis/index.html)
@@ -104,11 +229,12 @@ LightRAG full documentation available at [lightrag.sylph.ai](https://lightrag.sy
# Citation
```bibtex
-@software{Yin-LightRAG-2024,
- author = {Yin, Li},
- title = {{LightRAG: The PyTorch Library for Large language Model (LLM) Applications}},
+@software{Yin2024LightRAG,
+ author = {Li Yin},
+ title = {{LightRAG: The PyTorch Library for Large Language Model (LLM) Applications}},
month = {7},
year = {2024},
+ doi = {10.5281/zenodo.12639531},
url = {https://github.com/SylphAI-Inc/LightRAG}
}
```
diff --git a/benchmarks/ReAct_agent/fever/fever.py b/benchmarks/ReAct_agent/fever/fever.py
index 4564b5ee..1b0867cf 100644
--- a/benchmarks/ReAct_agent/fever/fever.py
+++ b/benchmarks/ReAct_agent/fever/fever.py
@@ -7,13 +7,13 @@
"""
import dotenv
-from components.api_client.openai_client import OpenAIClient
-from components.agent.react_agent import ReActAgent
-from core.func_tool import FunctionTool
-from components.api_client import GroqAPIClient
+from lightrag.components.model_client.openai_client import OpenAIClient
+from lightrag.components.agent.react import ReActAgent
+from lightrag.core.func_tool import FunctionTool
+from lightrag.components.model_client import GroqAPIClient
import time
from benchmarks.ReAct_agent.utils.tools import search, lookup, normalize_answer
-from eval.evaluators import AnswerMacthEvaluator
+from lightrag.eval.answer_match_acc import AnswerMatchAcc
import logging
import json
from typing import List, Optional, Any, Dict
@@ -229,8 +229,8 @@ def experiment(
# setup evaluators
-EM_evaluator = AnswerMacthEvaluator(type="exact_match")
-FM_evaluator = AnswerMacthEvaluator(type="fuzzy_match")
+EM_evaluator = AnswerMatchAcc(type="exact_match")
+FM_evaluator = AnswerMatchAcc(type="fuzzy_match")
# load test data
file = open("./tests/benchmark/ReAct_agent/paper_data/paper_dev_10.json")
diff --git a/class_hierarchy_edges.csv b/class_hierarchy_edges.csv
index a9348645..bbbcc098 100644
--- a/class_hierarchy_edges.csv
+++ b/class_hierarchy_edges.csv
@@ -1,3 +1,6 @@
+Component,BooleanParser
+Component,IntParser
+Component,FloatParser
Component,ListParser
Component,JsonParser
Component,YamlParser
@@ -15,7 +18,6 @@ Component,FunComponent
Component,ReActAgent
Component,OutputParser
Component,TextSplitter
-Component,DocumentSplitter
Component,ToEmbeddings
Component,RetrieverOutputToContextStr
Component,DefaultLLMJudge
@@ -56,8 +58,6 @@ DataClass,DialogTurn
DataClass,Instruction
DataClass,GeneratorStatesRecord
DataClass,GeneratorCallRecord
-Generator,CoTGenerator
-Generator,CoTGeneratorWithJsonOutput
OutputParser,YamlOutputParser
OutputParser,JsonOutputParser
OutputParser,ListOutputParser
diff --git a/developer_notes/__init__.py b/developer_notes/__init__.py
index d33bab7c..d4d88633 100644
--- a/developer_notes/__init__.py
+++ b/developer_notes/__init__.py
@@ -1,3 +1,4 @@
from lightrag.utils import setup_env
+print("initiating setup_env()...")
setup_env()
diff --git a/developer_notes/generator_note.py b/developer_notes/generator_note.py
index 2fa89251..f7a57f2e 100644
--- a/developer_notes/generator_note.py
+++ b/developer_notes/generator_note.py
@@ -1,5 +1,11 @@
-from lightrag.core import Component, Generator
+from dataclasses import dataclass, field
+
+from lightrag.core import Component, Generator, DataClass, fun_to_component, Sequential
from lightrag.components.model_client import GroqAPIClient
+from lightrag.components.output_parsers import JsonOutputParser
+from lightrag.utils import setup_env
+
+setup_env()
class SimpleQA(Component):
@@ -24,6 +30,47 @@ async def acall(self, query):
return await self.generator.acall({"input_str": query})
+@dataclass
+class QAOutput(DataClass):
+ explaination: str = field(
+ metadata={"desc": "A brief explaination of the concept in one sentence."}
+ )
+ example: str = field(metadata={"desc": "An example of the concept in a sentence."})
+
+
+@fun_to_component
+def to_qa_output(data: dict) -> QAOutput:
+ return QAOutput.from_dict(data)
+
+
+class QA(Component):
+ def __init__(self):
+ super().__init__()
+ template = r"""
+You are a helpful assistant.
+
+{{output_format_str}}
+
+
+User: {{input_str}}
+You:
+ """
+ parser = JsonOutputParser(data_class=QAOutput)
+ self.generator = Generator(
+ model_client=GroqAPIClient(),
+ model_kwargs={"model": "llama3-8b-8192"},
+ template=template,
+ prompt_kwargs={"output_format_str": parser.format_instructions()},
+ output_processors=Sequential(parser, to_qa_output),
+ )
+
+ def call(self, query: str):
+ return self.generator.call({"input_str": query})
+
+ async def acall(self, query: str):
+ return await self.generator.acall({"input_str": query})
+
+
def minimum_generator():
from lightrag.core import Generator
from lightrag.components.model_client import GroqAPIClient
@@ -75,8 +122,8 @@ def use_its_own_template():
from lightrag.components.model_client import GroqAPIClient
template = r"""{{task_desc_str}}
- User: {{input_str}}
- You:"""
+User: {{input_str}}
+You:"""
generator = Generator(
model_client=GroqAPIClient(),
model_kwargs={"model": "llama3-8b-8192"},
@@ -169,13 +216,22 @@ def create_purely_from_config_2():
if __name__ == "__main__":
- qa = SimpleQA()
- answer = qa("What is LightRAG?")
- print(qa)
-
- minimum_generator()
- use_a_json_parser()
- use_its_own_template()
- use_model_client_enum_to_switch_client()
- create_purely_from_config()
- create_purely_from_config_2()
+ qa1 = SimpleQA()
+ answer = qa1("What is LightRAG?")
+ print(qa1)
+
+ qa2 = QA()
+ answer = qa2("What is LLM?")
+ print(qa2)
+ print(answer)
+ qa2.generator.print_prompt(
+ output_format_str=qa2.generator.output_processors[0].format_instructions(),
+ input_str="What is LLM?",
+ )
+
+ # minimum_generator()
+ # use_a_json_parser()
+ # use_its_own_template()
+ # use_model_client_enum_to_switch_client()
+ # create_purely_from_config()
+ # create_purely_from_config_2()
diff --git a/docs/Makefile b/docs/Makefile
index b327c069..c57fd100 100644
--- a/docs/Makefile
+++ b/docs/Makefile
@@ -1,12 +1,10 @@
# Minimal makefile for Sphinx documentation
-#
-
# You can set these variables from the command line.
SPHINXOPTS =
SPHINXBUILD = sphinx-build
SPHINXPROJ = LightRAG
-SOURCEDIR = source# the source of output and conf.py
+SOURCEDIR = source
BUILDDIR = build
APIDOCOUTDIR = $(SOURCEDIR)/apis
@@ -28,6 +26,12 @@ apidoc:
@sphinx-apidoc -o $(APIDOCOUTDIR)/optim ../lightrag/lightrag/optim --separate --force
@sphinx-apidoc -o $(APIDOCOUTDIR)/utils ../lightrag/lightrag/utils --separate --force
@sphinx-apidoc -o $(APIDOCOUTDIR)/tracing ../lightrag/lightrag/tracing --separate --force
+
+generate_autosummary:
+ @echo "Generating autosummary files"
+ @sphinx-autogen $(SOURCEDIR)/**/*.rst
+
+update_files:
@echo "Inserting reference labels into RST files."
@python $(SOURCEDIR)/insert_labels.py
@echo "Removing unnecessary strings for better formatting"
@@ -36,9 +40,6 @@ apidoc:
@python $(SOURCEDIR)/remove_files.py
@echo "Renaming and updating file"
@python $(SOURCEDIR)/change_api_file_name.py
- # @echo "Renaming and updating file"
- # @python $(SOURCEDIR)/change_api_file_name_autosummary.py
-
-html: apidoc
+html: apidoc generate_autosummary update_files
@$(SPHINXBUILD) -b html "$(SOURCEDIR)" "$(BUILDDIR)" $(SPHINXOPTS) $(O)
diff --git a/docs/source/_static/class_hierarchy.html b/docs/source/_static/class_hierarchy.html
index f6f6256f..22e4202a 100644
--- a/docs/source/_static/class_hierarchy.html
+++ b/docs/source/_static/class_hierarchy.html
@@ -88,8 +88,8 @@
// parsing and collecting nodes and edges from the python
- nodes = new vis.DataSet([{"color": "#97c2fc", "id": "Component", "label": "Component", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ListParser", "label": "ListParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "JsonParser", "label": "JsonParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "YamlParser", "label": "YamlParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ToolManager", "label": "ToolManager", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Prompt", "label": "Prompt", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ModelClient", "label": "ModelClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Retriever", "label": "Retriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunctionTool", "label": "FunctionTool", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Tokenizer", "label": "Tokenizer", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Generator", "label": "Generator", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Embedder", "label": "Embedder", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BatchEmbedder", "label": "BatchEmbedder", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Sequential", "label": "Sequential", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunComponent", "label": "FunComponent", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ReActAgent", "label": "ReActAgent", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "OutputParser", "label": "OutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "TextSplitter", "label": "TextSplitter", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DocumentSplitter", "label": "DocumentSplitter", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ToEmbeddings", "label": "ToEmbeddings", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "RetrieverOutputToContextStr", "label": "RetrieverOutputToContextStr", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DefaultLLMJudge", "label": "DefaultLLMJudge", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "LLMAugmenter", "label": "LLMAugmenter", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "CohereAPIClient", "label": "CohereAPIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "TransformersClient", "label": "TransformersClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GroqAPIClient", "label": "GroqAPIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GoogleGenAIClient", "label": "GoogleGenAIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "OpenAIClient", "label": "OpenAIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "AnthropicAPIClient", "label": "AnthropicAPIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BM25Retriever", "label": "BM25Retriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "PostgresRetriever", "label": "PostgresRetriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "RerankerRetriever", "label": "RerankerRetriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "LLMRetriever", "label": "LLMRetriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FAISSRetriever", "label": "FAISSRetriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "CoTGenerator", "label": "CoTGenerator", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "CoTGeneratorWithJsonOutput", "label": "CoTGeneratorWithJsonOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "YamlOutputParser", "label": "YamlOutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "JsonOutputParser", "label": "JsonOutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ListOutputParser", "label": "ListOutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BooleanOutputParser", "label": "BooleanOutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Generic", "label": "Generic", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "LocalDB", "label": "LocalDB", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GeneratorOutput", "label": "GeneratorOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Parameter", "label": "Parameter", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Sample", "label": "Sample", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Sampler", "label": "Sampler", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "RandomSampler", "label": "RandomSampler", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ClassSampler", "label": "ClassSampler", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Enum", "label": "Enum", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DataClassFormatType", "label": "DataClassFormatType", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ModelType", "label": "ModelType", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DistanceToOperator", "label": "DistanceToOperator", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "OptionalPackages", "label": "OptionalPackages", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DataClass", "label": "DataClass", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "EmbedderOutput", "label": "EmbedderOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "RetrieverOutput", "label": "RetrieverOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunctionDefinition", "label": "FunctionDefinition", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Function", "label": "Function", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunctionExpression", "label": "FunctionExpression", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunctionOutput", "label": "FunctionOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "StepOutput", "label": "StepOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Document", "label": "Document", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DialogTurn", "label": "DialogTurn", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Instruction", "label": "Instruction", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GeneratorStatesRecord", "label": "GeneratorStatesRecord", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GeneratorCallRecord", "label": "GeneratorCallRecord", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Optimizer", "label": "Optimizer", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BootstrapFewShot", "label": "BootstrapFewShot", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "LLMOptimizer", "label": "LLMOptimizer", "shape": "dot", "size": 10}]);
- edges = new vis.DataSet([{"arrows": "to", "from": "Component", "to": "ListParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "JsonParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "YamlParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "ToolManager", "width": 1}, {"arrows": "to", "from": "Component", "to": "Prompt", "width": 1}, {"arrows": "to", "from": "Component", "to": "ModelClient", "width": 1}, {"arrows": "to", "from": "Component", "to": "Retriever", "width": 1}, {"arrows": "to", "from": "Component", "to": "FunctionTool", "width": 1}, {"arrows": "to", "from": "Component", "to": "Tokenizer", "width": 1}, {"arrows": "to", "from": "Component", "to": "Generator", "width": 1}, {"arrows": "to", "from": "Component", "to": "Embedder", "width": 1}, {"arrows": "to", "from": "Component", "to": "BatchEmbedder", "width": 1}, {"arrows": "to", "from": "Component", "to": "Sequential", "width": 1}, {"arrows": "to", "from": "Component", "to": "FunComponent", "width": 1}, {"arrows": "to", "from": "Component", "to": "ReActAgent", "width": 1}, {"arrows": "to", "from": "Component", "to": "OutputParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "TextSplitter", "width": 1}, {"arrows": "to", "from": "Component", "to": "DocumentSplitter", "width": 1}, {"arrows": "to", "from": "Component", "to": "ToEmbeddings", "width": 1}, {"arrows": "to", "from": "Component", "to": "RetrieverOutputToContextStr", "width": 1}, {"arrows": "to", "from": "Component", "to": "DefaultLLMJudge", "width": 1}, {"arrows": "to", "from": "Component", "to": "LLMAugmenter", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "CohereAPIClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "TransformersClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "GroqAPIClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "GoogleGenAIClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "OpenAIClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "AnthropicAPIClient", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "BM25Retriever", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "PostgresRetriever", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "RerankerRetriever", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "LLMRetriever", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "FAISSRetriever", "width": 1}, {"arrows": "to", "from": "Generator", "to": "CoTGenerator", "width": 1}, {"arrows": "to", "from": "Generator", "to": "CoTGeneratorWithJsonOutput", "width": 1}, {"arrows": "to", "from": "OutputParser", "to": "YamlOutputParser", "width": 1}, {"arrows": "to", "from": "OutputParser", "to": "JsonOutputParser", "width": 1}, {"arrows": "to", "from": "OutputParser", "to": "ListOutputParser", "width": 1}, {"arrows": "to", "from": "OutputParser", "to": "BooleanOutputParser", "width": 1}, {"arrows": "to", "from": "Generic", "to": "LocalDB", "width": 1}, {"arrows": "to", "from": "Generic", "to": "Retriever", "width": 1}, {"arrows": "to", "from": "Generic", "to": "GeneratorOutput", "width": 1}, {"arrows": "to", "from": "Generic", "to": "Parameter", "width": 1}, {"arrows": "to", "from": "Generic", "to": "Sample", "width": 1}, {"arrows": "to", "from": "Generic", "to": "Sampler", "width": 1}, {"arrows": "to", "from": "Generic", "to": "RandomSampler", "width": 1}, {"arrows": "to", "from": "Generic", "to": "ClassSampler", "width": 1}, {"arrows": "to", "from": "Sampler", "to": "RandomSampler", "width": 1}, {"arrows": "to", "from": "Sampler", "to": "ClassSampler", "width": 1}, {"arrows": "to", "from": "Enum", "to": "DataClassFormatType", "width": 1}, {"arrows": "to", "from": "Enum", "to": "ModelType", "width": 1}, {"arrows": "to", "from": "Enum", "to": "DistanceToOperator", "width": 1}, {"arrows": "to", "from": "Enum", "to": "OptionalPackages", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "EmbedderOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "GeneratorOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "RetrieverOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "FunctionDefinition", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "Function", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "FunctionExpression", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "FunctionOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "StepOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "Document", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "DialogTurn", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "Instruction", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "GeneratorStatesRecord", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "GeneratorCallRecord", "width": 1}, {"arrows": "to", "from": "Optimizer", "to": "BootstrapFewShot", "width": 1}, {"arrows": "to", "from": "Optimizer", "to": "LLMOptimizer", "width": 1}]);
+ nodes = new vis.DataSet([{"color": "#97c2fc", "id": "Component", "label": "Component", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BooleanParser", "label": "BooleanParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "IntParser", "label": "IntParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FloatParser", "label": "FloatParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ListParser", "label": "ListParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "JsonParser", "label": "JsonParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "YamlParser", "label": "YamlParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ToolManager", "label": "ToolManager", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Prompt", "label": "Prompt", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ModelClient", "label": "ModelClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Retriever", "label": "Retriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunctionTool", "label": "FunctionTool", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Tokenizer", "label": "Tokenizer", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Generator", "label": "Generator", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Embedder", "label": "Embedder", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BatchEmbedder", "label": "BatchEmbedder", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Sequential", "label": "Sequential", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunComponent", "label": "FunComponent", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ReActAgent", "label": "ReActAgent", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "OutputParser", "label": "OutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "TextSplitter", "label": "TextSplitter", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ToEmbeddings", "label": "ToEmbeddings", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "RetrieverOutputToContextStr", "label": "RetrieverOutputToContextStr", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DefaultLLMJudge", "label": "DefaultLLMJudge", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "LLMAugmenter", "label": "LLMAugmenter", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "CohereAPIClient", "label": "CohereAPIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "TransformersClient", "label": "TransformersClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GroqAPIClient", "label": "GroqAPIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GoogleGenAIClient", "label": "GoogleGenAIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "OpenAIClient", "label": "OpenAIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "AnthropicAPIClient", "label": "AnthropicAPIClient", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BM25Retriever", "label": "BM25Retriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "PostgresRetriever", "label": "PostgresRetriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "RerankerRetriever", "label": "RerankerRetriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "LLMRetriever", "label": "LLMRetriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FAISSRetriever", "label": "FAISSRetriever", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "YamlOutputParser", "label": "YamlOutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "JsonOutputParser", "label": "JsonOutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ListOutputParser", "label": "ListOutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BooleanOutputParser", "label": "BooleanOutputParser", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Sampler", "label": "Sampler", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "RandomSampler", "label": "RandomSampler", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ClassSampler", "label": "ClassSampler", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Enum", "label": "Enum", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DataClassFormatType", "label": "DataClassFormatType", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "ModelType", "label": "ModelType", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DistanceToOperator", "label": "DistanceToOperator", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "OptionalPackages", "label": "OptionalPackages", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DataClass", "label": "DataClass", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "EmbedderOutput", "label": "EmbedderOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GeneratorOutput", "label": "GeneratorOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "RetrieverOutput", "label": "RetrieverOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunctionDefinition", "label": "FunctionDefinition", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Function", "label": "Function", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunctionExpression", "label": "FunctionExpression", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "FunctionOutput", "label": "FunctionOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "StepOutput", "label": "StepOutput", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Document", "label": "Document", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "DialogTurn", "label": "DialogTurn", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Instruction", "label": "Instruction", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GeneratorStatesRecord", "label": "GeneratorStatesRecord", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "GeneratorCallRecord", "label": "GeneratorCallRecord", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Optimizer", "label": "Optimizer", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "BootstrapFewShot", "label": "BootstrapFewShot", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "LLMOptimizer", "label": "LLMOptimizer", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "LocalDB", "label": "LocalDB", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Parameter", "label": "Parameter", "shape": "dot", "size": 10}, {"color": "#97c2fc", "id": "Sample", "label": "Sample", "shape": "dot", "size": 10}]);
+ edges = new vis.DataSet([{"arrows": "to", "from": "Component", "to": "BooleanParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "IntParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "FloatParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "ListParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "JsonParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "YamlParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "ToolManager", "width": 1}, {"arrows": "to", "from": "Component", "to": "Prompt", "width": 1}, {"arrows": "to", "from": "Component", "to": "ModelClient", "width": 1}, {"arrows": "to", "from": "Component", "to": "Retriever", "width": 1}, {"arrows": "to", "from": "Component", "to": "FunctionTool", "width": 1}, {"arrows": "to", "from": "Component", "to": "Tokenizer", "width": 1}, {"arrows": "to", "from": "Component", "to": "Generator", "width": 1}, {"arrows": "to", "from": "Component", "to": "Embedder", "width": 1}, {"arrows": "to", "from": "Component", "to": "BatchEmbedder", "width": 1}, {"arrows": "to", "from": "Component", "to": "Sequential", "width": 1}, {"arrows": "to", "from": "Component", "to": "FunComponent", "width": 1}, {"arrows": "to", "from": "Component", "to": "ReActAgent", "width": 1}, {"arrows": "to", "from": "Component", "to": "OutputParser", "width": 1}, {"arrows": "to", "from": "Component", "to": "TextSplitter", "width": 1}, {"arrows": "to", "from": "Component", "to": "ToEmbeddings", "width": 1}, {"arrows": "to", "from": "Component", "to": "RetrieverOutputToContextStr", "width": 1}, {"arrows": "to", "from": "Component", "to": "DefaultLLMJudge", "width": 1}, {"arrows": "to", "from": "Component", "to": "LLMAugmenter", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "CohereAPIClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "TransformersClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "GroqAPIClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "GoogleGenAIClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "OpenAIClient", "width": 1}, {"arrows": "to", "from": "ModelClient", "to": "AnthropicAPIClient", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "BM25Retriever", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "PostgresRetriever", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "RerankerRetriever", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "LLMRetriever", "width": 1}, {"arrows": "to", "from": "Retriever", "to": "FAISSRetriever", "width": 1}, {"arrows": "to", "from": "OutputParser", "to": "YamlOutputParser", "width": 1}, {"arrows": "to", "from": "OutputParser", "to": "JsonOutputParser", "width": 1}, {"arrows": "to", "from": "OutputParser", "to": "ListOutputParser", "width": 1}, {"arrows": "to", "from": "OutputParser", "to": "BooleanOutputParser", "width": 1}, {"arrows": "to", "from": "Sampler", "to": "RandomSampler", "width": 1}, {"arrows": "to", "from": "Sampler", "to": "ClassSampler", "width": 1}, {"arrows": "to", "from": "Enum", "to": "DataClassFormatType", "width": 1}, {"arrows": "to", "from": "Enum", "to": "ModelType", "width": 1}, {"arrows": "to", "from": "Enum", "to": "DistanceToOperator", "width": 1}, {"arrows": "to", "from": "Enum", "to": "OptionalPackages", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "EmbedderOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "GeneratorOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "RetrieverOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "FunctionDefinition", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "Function", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "FunctionExpression", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "FunctionOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "StepOutput", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "Document", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "DialogTurn", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "Instruction", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "GeneratorStatesRecord", "width": 1}, {"arrows": "to", "from": "DataClass", "to": "GeneratorCallRecord", "width": 1}, {"arrows": "to", "from": "Optimizer", "to": "BootstrapFewShot", "width": 1}, {"arrows": "to", "from": "Optimizer", "to": "LLMOptimizer", "width": 1}]);
nodeColors = {};
allNodes = nodes.get({ returnType: "Object" });
diff --git a/docs/source/_static/css/custom.css b/docs/source/_static/css/custom.css
index a97701dd..70018085 100644
--- a/docs/source/_static/css/custom.css
+++ b/docs/source/_static/css/custom.css
@@ -23,6 +23,12 @@
.pre {
color: #0a7d91; /* Change the color of ```` blocks */
}
+/* hide on this page */
+/* #pst-page-navigation-heading-2 {
+ display: none;
+} */
+
+
/* .copyright {
text-align: center;
diff --git a/docs/source/_templates/package.rst_t b/docs/source/_templates/package.rst_t
index 25c8aa34..5e1bbdc4 100644
--- a/docs/source/_templates/package.rst_t
+++ b/docs/source/_templates/package.rst_t
@@ -23,10 +23,6 @@
.. py:module:: {{ pkgname }}
{% endif %}
-{%- if modulefirst and not is_namespace %}
-{{ automodule(pkgname, automodule_options) }}
-{% endif %}
-
{%- if subpackages %}
Subpackages
-----------
@@ -35,30 +31,23 @@ Subpackages
{% endif %}
{%- if submodules %}
-Submodules
+Overview
----------
+
.. autosummary::
:toctree: _autosummary
{% for submodule in submodules %}
{{ submodule }}
{% endfor %}
-
-{% if separatemodules %}
-{{ toctree(submodules) }}
-{% else %}
-{%- for submodule in submodules %}
-{% if show_headings %}
-{{- [submodule, "module"] | join(" ") | e | heading(2) }}
-{% endif %}
-{{ automodule(submodule, automodule_options) }}
-{% endfor %}
-{%- endif %}
{%- endif %}
-{%- if not modulefirst and not is_namespace %}
+{%- if not is_namespace %}
Module contents
---------------
-{{ automodule(pkgname, automodule_options) }}
+.. autosummary::
+ :toctree: _autosummary
+
+ {{ pkgname }}
{% endif %}
diff --git a/docs/source/apis/components/_autosummary/components.agent.react.rst b/docs/source/apis/components/_autosummary/components.agent.react.rst
index 9c0d4e93..a2b2e5d6 100644
--- a/docs/source/apis/components/_autosummary/components.agent.react.rst
+++ b/docs/source/apis/components/_autosummary/components.agent.react.rst
@@ -1,4 +1,6 @@
-components.agent.react
+.. _components-agent-react:
+
+react
======================
.. automodule:: components.agent.react
diff --git a/docs/source/apis/components/_autosummary/components.api_client.anthropic_client.rst b/docs/source/apis/components/_autosummary/components.api_client.anthropic_client.rst
deleted file mode 100644
index 31e030aa..00000000
--- a/docs/source/apis/components/_autosummary/components.api_client.anthropic_client.rst
+++ /dev/null
@@ -1,22 +0,0 @@
-.. _components-api_client-anthropic_client:
-
-components.api\_client.anthropic\_client
-========================================
-
-.. automodule:: components.api_client.anthropic_client
-
-
-
-
-
-
-
-
-
-
-
- .. rubric:: Classes
-
- .. autosummary::
-
- AnthropicAPIClient
diff --git a/docs/source/apis/components/_autosummary/components.api_client.google_client.rst b/docs/source/apis/components/_autosummary/components.api_client.google_client.rst
deleted file mode 100644
index 8da90bc6..00000000
--- a/docs/source/apis/components/_autosummary/components.api_client.google_client.rst
+++ /dev/null
@@ -1,22 +0,0 @@
-.. _components-api_client-google_client:
-
-components.api\_client.google\_client
-=====================================
-
-.. automodule:: components.api_client.google_client
-
-
-
-
-
-
-
-
-
-
-
- .. rubric:: Classes
-
- .. autosummary::
-
- GoogleGenAIClient
diff --git a/docs/source/apis/components/_autosummary/components.api_client.groq_client.rst b/docs/source/apis/components/_autosummary/components.api_client.groq_client.rst
deleted file mode 100644
index ccaab3bd..00000000
--- a/docs/source/apis/components/_autosummary/components.api_client.groq_client.rst
+++ /dev/null
@@ -1,22 +0,0 @@
-.. _components-api_client-groq_client:
-
-components.api\_client.groq\_client
-===================================
-
-.. automodule:: components.api_client.groq_client
-
-
-
-
-
-
-
-
-
-
-
- .. rubric:: Classes
-
- .. autosummary::
-
- GroqAPIClient
diff --git a/docs/source/apis/components/_autosummary/components.api_client.openai_client.rst b/docs/source/apis/components/_autosummary/components.api_client.openai_client.rst
deleted file mode 100644
index 4c14edec..00000000
--- a/docs/source/apis/components/_autosummary/components.api_client.openai_client.rst
+++ /dev/null
@@ -1,22 +0,0 @@
-.. _components-api_client-openai_client:
-
-components.api\_client.openai\_client
-=====================================
-
-.. automodule:: components.api_client.openai_client
-
-
-
-
-
-
-
-
-
-
-
- .. rubric:: Classes
-
- .. autosummary::
-
- OpenAIClient
diff --git a/docs/source/apis/components/_autosummary/components.api_client.transformers_client.rst b/docs/source/apis/components/_autosummary/components.api_client.transformers_client.rst
deleted file mode 100644
index 0398fa6f..00000000
--- a/docs/source/apis/components/_autosummary/components.api_client.transformers_client.rst
+++ /dev/null
@@ -1,29 +0,0 @@
-.. _components-api_client-transformers_client:
-
-components.api\_client.transformers\_client
-===========================================
-
-.. automodule:: components.api_client.transformers_client
-
-
-
-
-
-
-
- .. rubric:: Functions
-
- .. autosummary::
-
- average_pool
-
-
-
-
-
- .. rubric:: Classes
-
- .. autosummary::
-
- TransformerEmbedder
- TransformersClient
diff --git a/docs/source/apis/components/_autosummary/components.data_process.data_components.rst b/docs/source/apis/components/_autosummary/components.data_process.data_components.rst
index 4482fe53..4ce6e0f7 100644
--- a/docs/source/apis/components/_autosummary/components.data_process.data_components.rst
+++ b/docs/source/apis/components/_autosummary/components.data_process.data_components.rst
@@ -1,4 +1,6 @@
-components.data\_process.data\_components
+.. _components-data_process-data_components:
+
+data_components
=========================================
.. automodule:: components.data_process.data_components
diff --git a/docs/source/apis/components/_autosummary/components.data_process.document_splitter.rst b/docs/source/apis/components/_autosummary/components.data_process.document_splitter.rst
deleted file mode 100644
index 205e73fc..00000000
--- a/docs/source/apis/components/_autosummary/components.data_process.document_splitter.rst
+++ /dev/null
@@ -1,28 +0,0 @@
-.. _components-data_process-document_splitter:
-
-components.data\_process.document\_splitter
-===========================================
-
-.. automodule:: components.data_process.document_splitter
-
-
-
-
-
-
-
- .. rubric:: Functions
-
- .. autosummary::
-
- split_text_by_token_fn
-
-
-
-
-
- .. rubric:: Classes
-
- .. autosummary::
-
- DocumentSplitter
diff --git a/docs/source/apis/components/_autosummary/components.data_process.text_splitter.rst b/docs/source/apis/components/_autosummary/components.data_process.text_splitter.rst
index 63a89e71..20b75323 100644
--- a/docs/source/apis/components/_autosummary/components.data_process.text_splitter.rst
+++ b/docs/source/apis/components/_autosummary/components.data_process.text_splitter.rst
@@ -1,4 +1,6 @@
-components.data\_process.text\_splitter
+.. _components-data_process-text_splitter:
+
+text_splitter
=======================================
.. automodule:: components.data_process.text_splitter
diff --git a/docs/source/apis/components/_autosummary/components.memory.memory.rst b/docs/source/apis/components/_autosummary/components.memory.memory.rst
index 0338de4d..7cbd4143 100644
--- a/docs/source/apis/components/_autosummary/components.memory.memory.rst
+++ b/docs/source/apis/components/_autosummary/components.memory.memory.rst
@@ -1,4 +1,6 @@
-components.memory.memory
+.. _components-memory-memory:
+
+memory
========================
.. automodule:: components.memory.memory
diff --git a/docs/source/apis/components/_autosummary/components.model_client.anthropic_client.rst b/docs/source/apis/components/_autosummary/components.model_client.anthropic_client.rst
index 3c6b45a7..61c84d05 100644
--- a/docs/source/apis/components/_autosummary/components.model_client.anthropic_client.rst
+++ b/docs/source/apis/components/_autosummary/components.model_client.anthropic_client.rst
@@ -1,4 +1,6 @@
-components.model\_client.anthropic\_client
+.. _components-model_client-anthropic_client:
+
+anthropic_client
==========================================
.. automodule:: components.model_client.anthropic_client
diff --git a/docs/source/apis/components/_autosummary/components.model_client.cohere_client.rst b/docs/source/apis/components/_autosummary/components.model_client.cohere_client.rst
index 03c29e46..73263e7d 100644
--- a/docs/source/apis/components/_autosummary/components.model_client.cohere_client.rst
+++ b/docs/source/apis/components/_autosummary/components.model_client.cohere_client.rst
@@ -1,4 +1,6 @@
-components.model\_client.cohere\_client
+.. _components-model_client-cohere_client:
+
+cohere_client
=======================================
.. automodule:: components.model_client.cohere_client
diff --git a/docs/source/apis/components/_autosummary/components.model_client.google_client.rst b/docs/source/apis/components/_autosummary/components.model_client.google_client.rst
deleted file mode 100644
index 744dc3bc..00000000
--- a/docs/source/apis/components/_autosummary/components.model_client.google_client.rst
+++ /dev/null
@@ -1,22 +0,0 @@
-.. _components-model_client-google_client:
-
-components.model\_client.google\_client
-=======================================
-
-.. automodule:: components.model_client.google_client
-
-
-
-
-
-
-
-
-
-
-
- .. rubric:: Classes
-
- .. autosummary::
-
- GoogleGenAIClient
diff --git a/docs/source/apis/components/_autosummary/components.model_client.groq_client.rst b/docs/source/apis/components/_autosummary/components.model_client.groq_client.rst
index 02650f40..789c0e73 100644
--- a/docs/source/apis/components/_autosummary/components.model_client.groq_client.rst
+++ b/docs/source/apis/components/_autosummary/components.model_client.groq_client.rst
@@ -1,4 +1,6 @@
-components.model\_client.groq\_client
+.. _components-model_client-groq_client:
+
+groq_client
=====================================
.. automodule:: components.model_client.groq_client
diff --git a/docs/source/apis/components/_autosummary/components.model_client.openai_client.rst b/docs/source/apis/components/_autosummary/components.model_client.openai_client.rst
index 9b8bc7e7..ae941be4 100644
--- a/docs/source/apis/components/_autosummary/components.model_client.openai_client.rst
+++ b/docs/source/apis/components/_autosummary/components.model_client.openai_client.rst
@@ -1,4 +1,6 @@
-components.model\_client.openai\_client
+.. _components-model_client-openai_client:
+
+openai_client
=======================================
.. automodule:: components.model_client.openai_client
diff --git a/docs/source/apis/components/_autosummary/components.model_client.transformers_client.rst b/docs/source/apis/components/_autosummary/components.model_client.transformers_client.rst
index cb1c9d33..6311637b 100644
--- a/docs/source/apis/components/_autosummary/components.model_client.transformers_client.rst
+++ b/docs/source/apis/components/_autosummary/components.model_client.transformers_client.rst
@@ -1,4 +1,6 @@
-components.model\_client.transformers\_client
+.. _components-model_client-transformers_client:
+
+transformers_client
=============================================
.. automodule:: components.model_client.transformers_client
diff --git a/docs/source/apis/components/_autosummary/components.model_client.utils.rst b/docs/source/apis/components/_autosummary/components.model_client.utils.rst
index 7d7f919e..55b7bb50 100644
--- a/docs/source/apis/components/_autosummary/components.model_client.utils.rst
+++ b/docs/source/apis/components/_autosummary/components.model_client.utils.rst
@@ -1,4 +1,6 @@
-components.model\_client.utils
+.. _components-model_client-utils:
+
+utils
==============================
.. automodule:: components.model_client.utils
diff --git a/docs/source/apis/components/_autosummary/components.output_parsers.outputs.rst b/docs/source/apis/components/_autosummary/components.output_parsers.outputs.rst
index 797976da..29de8634 100644
--- a/docs/source/apis/components/_autosummary/components.output_parsers.outputs.rst
+++ b/docs/source/apis/components/_autosummary/components.output_parsers.outputs.rst
@@ -1,4 +1,6 @@
-components.output\_parsers.outputs
+.. _components-output_parsers-outputs:
+
+outputs
==================================
.. automodule:: components.output_parsers.outputs
diff --git a/docs/source/apis/components/_autosummary/components.reasoning.chain_of_thought.rst b/docs/source/apis/components/_autosummary/components.reasoning.chain_of_thought.rst
index 665486a7..4ec1269c 100644
--- a/docs/source/apis/components/_autosummary/components.reasoning.chain_of_thought.rst
+++ b/docs/source/apis/components/_autosummary/components.reasoning.chain_of_thought.rst
@@ -1,4 +1,6 @@
-components.reasoning.chain\_of\_thought
+.. _components-reasoning-chain_of_thought:
+
+chain_of_thought
=======================================
.. automodule:: components.reasoning.chain_of_thought
diff --git a/docs/source/apis/components/_autosummary/components.retriever.bm25_retriever.rst b/docs/source/apis/components/_autosummary/components.retriever.bm25_retriever.rst
index 6f869f55..dcff5d44 100644
--- a/docs/source/apis/components/_autosummary/components.retriever.bm25_retriever.rst
+++ b/docs/source/apis/components/_autosummary/components.retriever.bm25_retriever.rst
@@ -1,4 +1,6 @@
-components.retriever.bm25\_retriever
+.. _components-retriever-bm25_retriever:
+
+bm25_retriever
====================================
.. automodule:: components.retriever.bm25_retriever
diff --git a/docs/source/apis/components/_autosummary/components.retriever.faiss_retriever.rst b/docs/source/apis/components/_autosummary/components.retriever.faiss_retriever.rst
index cad15914..2f972f21 100644
--- a/docs/source/apis/components/_autosummary/components.retriever.faiss_retriever.rst
+++ b/docs/source/apis/components/_autosummary/components.retriever.faiss_retriever.rst
@@ -1,4 +1,6 @@
-components.retriever.faiss\_retriever
+.. _components-retriever-faiss_retriever:
+
+faiss_retriever
=====================================
.. automodule:: components.retriever.faiss_retriever
diff --git a/docs/source/apis/components/_autosummary/components.retriever.llm_retriever.rst b/docs/source/apis/components/_autosummary/components.retriever.llm_retriever.rst
index d8c9c6c6..b5acd12c 100644
--- a/docs/source/apis/components/_autosummary/components.retriever.llm_retriever.rst
+++ b/docs/source/apis/components/_autosummary/components.retriever.llm_retriever.rst
@@ -1,4 +1,6 @@
-components.retriever.llm\_retriever
+.. _components-retriever-llm_retriever:
+
+llm_retriever
===================================
.. automodule:: components.retriever.llm_retriever
diff --git a/docs/source/apis/components/_autosummary/components.retriever.postgres_retriever.rst b/docs/source/apis/components/_autosummary/components.retriever.postgres_retriever.rst
deleted file mode 100644
index 6a844203..00000000
--- a/docs/source/apis/components/_autosummary/components.retriever.postgres_retriever.rst
+++ /dev/null
@@ -1,23 +0,0 @@
-.. _components-retriever-postgres_retriever:
-
-components.retriever.postgres\_retriever
-========================================
-
-.. automodule:: components.retriever.postgres_retriever
-
-
-
-
-
-
-
-
-
-
-
- .. rubric:: Classes
-
- .. autosummary::
-
- DistanceToOperator
- PostgresRetriever
diff --git a/docs/source/apis/components/_autosummary/components.retriever.reranker_retriever.rst b/docs/source/apis/components/_autosummary/components.retriever.reranker_retriever.rst
index 3231d4a6..4acf28a7 100644
--- a/docs/source/apis/components/_autosummary/components.retriever.reranker_retriever.rst
+++ b/docs/source/apis/components/_autosummary/components.retriever.reranker_retriever.rst
@@ -1,4 +1,6 @@
-components.retriever.reranker\_retriever
+.. _components-retriever-reranker_retriever:
+
+reranker_retriever
========================================
.. automodule:: components.retriever.reranker_retriever
diff --git a/docs/source/apis/components/agent_prompt.rst b/docs/source/apis/components/agent_prompt.rst
deleted file mode 100644
index 5432c912..00000000
--- a/docs/source/apis/components/agent_prompt.rst
+++ /dev/null
@@ -1,71 +0,0 @@
-.. _agent_prompt:
-
-.. _DEFAULT_REACT_AGENT_SYSTEM_PROMPT:
-
-DEFAULT_REACT_AGENT_SYSTEM_PROMPT
-----------------------------------
-
-This is the default prompt used by the system to interact with the agents. It contains the following structure:
-
-.. code-block:: python
-
- DEFAULT_REACT_AGENT_SYSTEM_PROMPT = r"""
- {# role/task description #}
- You task is to answer user's query with minimum steps and maximum accuracy using the tools provided.
- {# REACT instructions #}
- Each step you will read the previous Thought, Action, and Observation(execution result of the action)steps and then provide the next Thought and Action.
-
- You only have access to the following tools:
- {# tools #}
- {% for tool in tools %}
- {{ loop.index }}. ToolName: {{ tool.metadata.name }}
- Tool Description: {{ tool.metadata.description }}
- Tool Parameters: {{ tool.metadata.fn_schema_str }} {#tool args can be misleading, especially if we already have type hints and docstring in the function#}
- {% endfor %}
- {# output is always more robust to use json than string #}
- ---
- Your output must be in valid JSON format(raw Python string format) with two keys:
- {
- "thought": "",
- "action": "ToolName(, )"
- }
- - Must double quote the JSON str.
- - Inside of the JSON str, Must use escape double quote and escape backslash for string.
- For example:
- "action": "finish(\"John's.\")"
- ---
- {# Specifications TODO: preference between the usage of llm tool vs the other tool #}
- Process:
- - Step 1: Read the user query and potentially divide it into subqueries. And get started with the first subquery.
- - Call one available tool at a time to solve each subquery/subquestion. \
- - At step 'finish', join all subqueries answers and finish the task.
- Remember:
- - Action must call one of the above tools with Took Name. It can not be empty.
- - Read the Tool Description and ensure your args and kwarg follow what each tool expects in types. e.g. (a=1, b=2) if it is keyword argument or (1, 2) if it is positional.
- - You will always end with 'finish' action to finish the task. The answer can be the final answer or failure message.
- - When the initial query is simple, use minimum steps to answer the query.
- {#Examples can be here#}
- {# Check if there are any examples #}
- {% if examples %}
-
- {% for example in examples %}
- {{ example }}
- {% endfor %}
-
- {% endif %}
- <>
- -----------------
- {# History #}
- {% for history in step_history %}
- Step {{history.step}}:
- {
- "thought": "{{history.thought}}",
- "action": "{{history.action}}",
- }
- "observation": "{{history.observation}}"
- {% endfor %}
- {% if input_str %}
- User query:
- {{ input_str }}
- {% endif %}
- """
\ No newline at end of file
diff --git a/docs/source/apis/components/components.agent.rst b/docs/source/apis/components/components.agent.rst
index fde2b8a2..9e2fdcc7 100644
--- a/docs/source/apis/components/components.agent.rst
+++ b/docs/source/apis/components/components.agent.rst
@@ -3,8 +3,9 @@
agent
========================
-Submodules
+Overview
----------
+
.. autosummary::
:toctree: _autosummary
@@ -12,16 +13,9 @@ Submodules
components.agent.react
-
-.. toctree::
- :maxdepth: 4
-
- components.agent.react
-
-
---------------
-.. automodule:: components.agent
- :members:
- :undoc-members:
- :show-inheritance:
+.. autosummary::
+ :toctree: _autosummary
+
+ components.agent
diff --git a/docs/source/apis/components/components.data_process.document_splitter.rst b/docs/source/apis/components/components.data_process.document_splitter.rst
deleted file mode 100644
index 06832c3a..00000000
--- a/docs/source/apis/components/components.data_process.document_splitter.rst
+++ /dev/null
@@ -1,9 +0,0 @@
-.. _components-data_process-document_splitter:
-
-document\_splitter
-==================================================
-
-.. automodule:: components.data_process.document_splitter
- :members:
- :undoc-members:
- :show-inheritance:
diff --git a/docs/source/apis/components/components.data_process.rst b/docs/source/apis/components/components.data_process.rst
index 72ff7ae3..19875935 100644
--- a/docs/source/apis/components/components.data_process.rst
+++ b/docs/source/apis/components/components.data_process.rst
@@ -1,10 +1,11 @@
.. _components-data_process:
-data\_process
+data_process
================================
-Submodules
+Overview
----------
+
.. autosummary::
:toctree: _autosummary
@@ -14,17 +15,9 @@ Submodules
components.data_process.text_splitter
-
-.. toctree::
- :maxdepth: 4
-
- components.data_process.data_components
- components.data_process.text_splitter
-
-
---------------
-.. automodule:: components.data_process
- :members:
- :undoc-members:
- :show-inheritance:
+.. autosummary::
+ :toctree: _autosummary
+
+ components.data_process
diff --git a/docs/source/apis/components/components.memory.rst b/docs/source/apis/components/components.memory.rst
index 68fef512..4849cae4 100644
--- a/docs/source/apis/components/components.memory.rst
+++ b/docs/source/apis/components/components.memory.rst
@@ -3,8 +3,9 @@
memory
=========================
-Submodules
+Overview
----------
+
.. autosummary::
:toctree: _autosummary
@@ -12,16 +13,9 @@ Submodules
components.memory.memory
-
-.. toctree::
- :maxdepth: 4
-
- components.memory.memory
-
-
---------------
-.. automodule:: components.memory
- :members:
- :undoc-members:
- :show-inheritance:
+.. autosummary::
+ :toctree: _autosummary
+
+ components.memory
diff --git a/docs/source/apis/components/components.model_client.rst b/docs/source/apis/components/components.model_client.rst
index 57c78622..02644f25 100644
--- a/docs/source/apis/components/components.model_client.rst
+++ b/docs/source/apis/components/components.model_client.rst
@@ -1,10 +1,11 @@
.. _components-model_client:
-model\_client
+model_client
================================
-Submodules
+Overview
----------
+
.. autosummary::
:toctree: _autosummary
@@ -24,22 +25,9 @@ Submodules
components.model_client.utils
-
-.. toctree::
- :maxdepth: 4
-
- components.model_client.anthropic_client
- components.model_client.cohere_client
- components.model_client.google_client
- components.model_client.groq_client
- components.model_client.openai_client
- components.model_client.transformers_client
- components.model_client.utils
-
-
---------------
-.. automodule:: components.model_client
- :members:
- :undoc-members:
- :show-inheritance:
+.. autosummary::
+ :toctree: _autosummary
+
+ components.model_client
diff --git a/docs/source/apis/components/components.output_parsers.rst b/docs/source/apis/components/components.output_parsers.rst
index 2810e80c..12240889 100644
--- a/docs/source/apis/components/components.output_parsers.rst
+++ b/docs/source/apis/components/components.output_parsers.rst
@@ -1,10 +1,11 @@
.. _components-output_parsers:
-output\_parsers
+output_parsers
==================================
-Submodules
+Overview
----------
+
.. autosummary::
:toctree: _autosummary
@@ -12,16 +13,9 @@ Submodules
components.output_parsers.outputs
-
-.. toctree::
- :maxdepth: 4
-
- components.output_parsers.outputs
-
-
---------------
-.. automodule:: components.output_parsers
- :members:
- :undoc-members:
- :show-inheritance:
+.. autosummary::
+ :toctree: _autosummary
+
+ components.output_parsers
diff --git a/docs/source/apis/components/components.reasoning.rst b/docs/source/apis/components/components.reasoning.rst
index b17e5112..d53839c9 100644
--- a/docs/source/apis/components/components.reasoning.rst
+++ b/docs/source/apis/components/components.reasoning.rst
@@ -3,8 +3,9 @@
reasoning
============================
-Submodules
+Overview
----------
+
.. autosummary::
:toctree: _autosummary
@@ -12,16 +13,9 @@ Submodules
components.reasoning.chain_of_thought
-
-.. toctree::
- :maxdepth: 4
-
- components.reasoning.chain_of_thought
-
-
---------------
-.. automodule:: components.reasoning
- :members:
- :undoc-members:
- :show-inheritance:
+.. autosummary::
+ :toctree: _autosummary
+
+ components.reasoning
diff --git a/docs/source/apis/components/components.retriever.rst b/docs/source/apis/components/components.retriever.rst
index 27c507de..58e64386 100644
--- a/docs/source/apis/components/components.retriever.rst
+++ b/docs/source/apis/components/components.retriever.rst
@@ -3,8 +3,9 @@
retriever
============================
-Submodules
+Overview
----------
+
.. autosummary::
:toctree: _autosummary
@@ -20,20 +21,9 @@ Submodules
components.retriever.reranker_retriever
-
-.. toctree::
- :maxdepth: 4
-
- components.retriever.bm25_retriever
- components.retriever.faiss_retriever
- components.retriever.llm_retriever
- components.retriever.postgres_retriever
- components.retriever.reranker_retriever
-
-
---------------
-.. automodule:: components.retriever
- :members:
- :undoc-members:
- :show-inheritance:
+.. autosummary::
+ :toctree: _autosummary
+
+ components.retriever
diff --git a/docs/source/apis/components/index.rst b/docs/source/apis/components/index.rst
index 25ba56d9..2f414ef4 100644
--- a/docs/source/apis/components/index.rst
+++ b/docs/source/apis/components/index.rst
@@ -88,4 +88,4 @@ Reasoning
components.agent
components.data_process
components.reasoning
- components.memory
\ No newline at end of file
+ components.memory
diff --git a/docs/source/apis/core/core.base_data_class.rst b/docs/source/apis/core/core.base_data_class.rst
index 30fbfd14..6560da5b 100644
--- a/docs/source/apis/core/core.base_data_class.rst
+++ b/docs/source/apis/core/core.base_data_class.rst
@@ -1,6 +1,6 @@
.. _core-base_data_class:
-base\_data\_class
+base_data_class
=============================
.. automodule:: core.base_data_class
diff --git a/docs/source/apis/core/core.data_components.rst b/docs/source/apis/core/core.data_components.rst
deleted file mode 100644
index f3deeb8a..00000000
--- a/docs/source/apis/core/core.data_components.rst
+++ /dev/null
@@ -1,9 +0,0 @@
-.. _core-data_components:
-
-data\_components
-============================
-
-.. automodule:: core.data_components
- :members:
- :undoc-members:
- :show-inheritance:
diff --git a/docs/source/apis/core/core.default_prompt_template.rst b/docs/source/apis/core/core.default_prompt_template.rst
index 522ad037..7dc92b03 100644
--- a/docs/source/apis/core/core.default_prompt_template.rst
+++ b/docs/source/apis/core/core.default_prompt_template.rst
@@ -1,6 +1,6 @@
.. _core-default_prompt_template:
-default\_prompt\_template
+default_prompt_template
=====================================
.. automodule:: core.default_prompt_template
diff --git a/docs/source/apis/core/core.document_splitter.rst b/docs/source/apis/core/core.document_splitter.rst
deleted file mode 100644
index 77672d83..00000000
--- a/docs/source/apis/core/core.document_splitter.rst
+++ /dev/null
@@ -1,9 +0,0 @@
-.. _core-document_splitter:
-
-document\_splitter
-==============================
-
-.. automodule:: core.document_splitter
- :members:
- :undoc-members:
- :show-inheritance:
diff --git a/docs/source/apis/core/core.func_tool.rst b/docs/source/apis/core/core.func_tool.rst
index f8ab69fc..90707294 100644
--- a/docs/source/apis/core/core.func_tool.rst
+++ b/docs/source/apis/core/core.func_tool.rst
@@ -1,6 +1,6 @@
.. _core-func_tool:
-func\_tool
+func_tool
======================
.. automodule:: core.func_tool
diff --git a/docs/source/apis/core/core.memory.rst b/docs/source/apis/core/core.memory.rst
deleted file mode 100644
index 00e3de26..00000000
--- a/docs/source/apis/core/core.memory.rst
+++ /dev/null
@@ -1,9 +0,0 @@
-.. _core-memory:
-
-memory
-==================
-
-.. automodule:: core.memory
- :members:
- :undoc-members:
- :show-inheritance:
diff --git a/docs/source/apis/core/core.model_client.rst b/docs/source/apis/core/core.model_client.rst
index 4a61fd5e..5029f6e6 100644
--- a/docs/source/apis/core/core.model_client.rst
+++ b/docs/source/apis/core/core.model_client.rst
@@ -1,6 +1,6 @@
.. _core-model_client:
-model\_client
+model_client
=========================
.. automodule:: core.model_client
diff --git a/docs/source/apis/core/core.prompt_builder.rst b/docs/source/apis/core/core.prompt_builder.rst
index 26c80b01..8545afa9 100644
--- a/docs/source/apis/core/core.prompt_builder.rst
+++ b/docs/source/apis/core/core.prompt_builder.rst
@@ -1,6 +1,6 @@
.. _core-prompt_builder:
-prompt\_builder
+prompt_builder
===========================
.. automodule:: core.prompt_builder
diff --git a/docs/source/apis/core/core.string_parser.rst b/docs/source/apis/core/core.string_parser.rst
index 14d2a06e..3553cd59 100644
--- a/docs/source/apis/core/core.string_parser.rst
+++ b/docs/source/apis/core/core.string_parser.rst
@@ -1,6 +1,6 @@
.. _core-string_parser:
-string\_parser
+string_parser
==========================
.. automodule:: core.string_parser
diff --git a/docs/source/apis/core/core.tool_manager.rst b/docs/source/apis/core/core.tool_manager.rst
index 997401a8..501c3630 100644
--- a/docs/source/apis/core/core.tool_manager.rst
+++ b/docs/source/apis/core/core.tool_manager.rst
@@ -1,6 +1,6 @@
.. _core-tool_manager:
-tool\_manager
+tool_manager
=========================
.. automodule:: core.tool_manager
diff --git a/docs/source/apis/core/index.rst b/docs/source/apis/core/index.rst
index c9f7399b..5a929d47 100644
--- a/docs/source/apis/core/index.rst
+++ b/docs/source/apis/core/index.rst
@@ -3,7 +3,10 @@
Core
===================
-The core section of the LightRAG API documentation provides detailed information about the foundational components of the LightRAG system. These components are essential for the basic operations and serve as the building blocks for higher-level functionalities.
+.. The core section of the LightRAG API documentation provides detailed information about the foundational components of the LightRAG system. These components are essential for the basic operations and serve as the building blocks for higher-level functionalities.
+
+Core building blocks for RAG and more advanced functionalities, such as agents.
+
Overview
----------
@@ -11,91 +14,41 @@ Overview
core.base_data_class
core.component
- core.db
core.default_prompt_template
core.embedder
- core.functional
core.generator
- core.memory
core.model_client
- core.parameter
core.prompt_builder
core.retriever
core.string_parser
- core.tokenizer
core.func_tool
core.tool_manager
core.types
+ core.db
+ core.functional
+ core.parameter
+ core.tokenizer
-Model Client
----------------
-.. toctree::
- :maxdepth: 1
-
- core.model_client
-
-Component
---------------
-.. toctree::
- :maxdepth: 1
- core.component
-Data Handling
--------------
.. toctree::
:maxdepth: 1
+ :hidden:
core.base_data_class
- core.types
-
- core.db
-
-Prompts and Templates
----------------------
-.. toctree::
- :maxdepth: 1
-
+ core.component
core.default_prompt_template
- core.prompt_builder
-
-.. Document Processing
-.. -------------------
-.. .. toctree::
-.. :maxdepth: 1
-
- .. core.document_splitter
- core.text_splitter
-
-Embedding and Retrieval
------------------------
-.. toctree::
- :maxdepth: 1
-
core.embedder
- core.retriever
-
-Generation and Utilities
-------------------------
-.. toctree::
- :maxdepth: 1
-
core.generator
- core.functional
- core.memory
-
-------------------------
-.. toctree::
- :maxdepth: 1
-
+ core.model_client
+ core.prompt_builder
+ core.retriever
core.string_parser
- core.tokenizer
core.func_tool
-
-Parameters
-------------------------
-.. toctree::
- :maxdepth: 1
-
+ core.tool_manager
+ core.types
+ core.db
+ core.functional
core.parameter
+ core.tokenizer
diff --git a/docs/source/apis/eval/eval.answer_match_acc.rst b/docs/source/apis/eval/eval.answer_match_acc.rst
index b6a0e05b..8d16f6fe 100644
--- a/docs/source/apis/eval/eval.answer_match_acc.rst
+++ b/docs/source/apis/eval/eval.answer_match_acc.rst
@@ -1,6 +1,6 @@
.. _eval-answer_match_acc:
-answer\_match\_acc
+answer_match_acc
==============================
.. automodule:: eval.answer_match_acc
diff --git a/docs/source/apis/eval/eval.evaluators.rst b/docs/source/apis/eval/eval.evaluators.rst
index ac0de161..76e0225b 100644
--- a/docs/source/apis/eval/eval.evaluators.rst
+++ b/docs/source/apis/eval/eval.evaluators.rst
@@ -1,4 +1,4 @@
-.. _eval-evaluators:
+_eval-evaluators:
evaluators
======================
diff --git a/docs/source/apis/eval/eval.llm_as_judge.rst b/docs/source/apis/eval/eval.llm_as_judge.rst
index 77774ed3..1c4c426e 100644
--- a/docs/source/apis/eval/eval.llm_as_judge.rst
+++ b/docs/source/apis/eval/eval.llm_as_judge.rst
@@ -1,6 +1,6 @@
.. _eval-llm_as_judge:
-llm\_as\_judge
+llm_as_judge
==========================
.. automodule:: eval.llm_as_judge
diff --git a/docs/source/apis/eval/eval.retriever_recall.rst b/docs/source/apis/eval/eval.retriever_recall.rst
index 1eade14a..c087d08f 100644
--- a/docs/source/apis/eval/eval.retriever_recall.rst
+++ b/docs/source/apis/eval/eval.retriever_recall.rst
@@ -1,6 +1,6 @@
.. _eval-retriever_recall:
-retriever\_recall
+retriever_recall
=============================
.. automodule:: eval.retriever_recall
diff --git a/docs/source/apis/eval/eval.retriever_relevance.rst b/docs/source/apis/eval/eval.retriever_relevance.rst
index bd2c721b..c7af1274 100644
--- a/docs/source/apis/eval/eval.retriever_relevance.rst
+++ b/docs/source/apis/eval/eval.retriever_relevance.rst
@@ -1,6 +1,6 @@
.. _eval-retriever_relevance:
-retriever\_relevance
+retriever_relevance
================================
.. automodule:: eval.retriever_relevance
diff --git a/docs/source/apis/eval/index.rst b/docs/source/apis/eval/index.rst
index d3b09e39..afe06e45 100644
--- a/docs/source/apis/eval/index.rst
+++ b/docs/source/apis/eval/index.rst
@@ -12,10 +12,10 @@ Overview
eval.retriever_relevance
eval.llm_as_judge
-Evaluator
-----------
+
.. toctree::
:maxdepth: 1
+ :hidden:
eval.answer_match_acc
eval.retriever_recall
diff --git a/docs/source/apis/optim/optim.few_shot_optimizer.rst b/docs/source/apis/optim/optim.few_shot_optimizer.rst
index d411e628..18cc2e3e 100644
--- a/docs/source/apis/optim/optim.few_shot_optimizer.rst
+++ b/docs/source/apis/optim/optim.few_shot_optimizer.rst
@@ -1,6 +1,6 @@
.. _optim-few_shot_optimizer:
-few\_shot\_optimizer
+few_shot_optimizer
=================================
.. automodule:: optim.few_shot_optimizer
diff --git a/docs/source/apis/optim/optim.llm_augment.rst b/docs/source/apis/optim/optim.llm_augment.rst
index 44ff64d7..f3626278 100644
--- a/docs/source/apis/optim/optim.llm_augment.rst
+++ b/docs/source/apis/optim/optim.llm_augment.rst
@@ -1,6 +1,6 @@
.. _optim-llm_augment:
-llm\_augment
+llm_augment
=========================
.. automodule:: optim.llm_augment
diff --git a/docs/source/apis/optim/optim.llm_optimizer.rst b/docs/source/apis/optim/optim.llm_optimizer.rst
index 8c27727a..08ee2cc8 100644
--- a/docs/source/apis/optim/optim.llm_optimizer.rst
+++ b/docs/source/apis/optim/optim.llm_optimizer.rst
@@ -1,6 +1,6 @@
.. _optim-llm_optimizer:
-llm\_optimizer
+llm_optimizer
===========================
.. automodule:: optim.llm_optimizer
diff --git a/docs/source/apis/tracing/index.rst b/docs/source/apis/tracing/index.rst
index 7a266ecd..13f7add5 100644
--- a/docs/source/apis/tracing/index.rst
+++ b/docs/source/apis/tracing/index.rst
@@ -11,17 +11,11 @@ Overview
tracing.generator_state_logger
tracing.generator_call_logger
-Decorators
---------------
-.. toctree::
- :maxdepth: 1
- tracing.decorators
-
-Loggers
---------------
.. toctree::
:maxdepth: 1
+ :hidden:
+ tracing.decorators
tracing.generator_state_logger
tracing.generator_call_logger
diff --git a/docs/source/apis/tracing/tracing.generator_call_logger.rst b/docs/source/apis/tracing/tracing.generator_call_logger.rst
index 80578c48..bb546cc4 100644
--- a/docs/source/apis/tracing/tracing.generator_call_logger.rst
+++ b/docs/source/apis/tracing/tracing.generator_call_logger.rst
@@ -1,6 +1,6 @@
.. _tracing-generator_call_logger:
-generator\_call\_logger
+generator_call_logger
======================================
.. automodule:: tracing.generator_call_logger
diff --git a/docs/source/apis/tracing/tracing.generator_state_logger.rst b/docs/source/apis/tracing/tracing.generator_state_logger.rst
index 4a2bfa34..e0f1caed 100644
--- a/docs/source/apis/tracing/tracing.generator_state_logger.rst
+++ b/docs/source/apis/tracing/tracing.generator_state_logger.rst
@@ -1,6 +1,6 @@
.. _tracing-generator_state_logger:
-generator\_state\_logger
+generator_state_logger
=======================================
.. automodule:: tracing.generator_state_logger
diff --git a/docs/source/apis/utils/index.rst b/docs/source/apis/utils/index.rst
index fb339773..728a7338 100644
--- a/docs/source/apis/utils/index.rst
+++ b/docs/source/apis/utils/index.rst
@@ -12,24 +12,15 @@ Overview
utils.config
utils.registry
utils.setup_env
+ utils.lazy_import
-Logger
---------
.. toctree::
:maxdepth: 1
+ :hidden:
utils.logger
-
-Serialization
-----------------
-.. toctree::
- :maxdepth: 1
-
utils.serialization
-
-Setup_env
---------------
-.. toctree::
- :maxdepth: 1
-
+ utils.config
+ utils.registry
utils.setup_env
+ utils.lazy_import
diff --git a/docs/source/apis/utils/utils.file_io.rst b/docs/source/apis/utils/utils.file_io.rst
index a6bd2610..1dfb7a95 100644
--- a/docs/source/apis/utils/utils.file_io.rst
+++ b/docs/source/apis/utils/utils.file_io.rst
@@ -1,6 +1,6 @@
.. _utils-file_io:
-file\_io
+file_io
=====================
.. automodule:: utils.file_io
diff --git a/docs/source/apis/utils/utils.lazy_import.rst b/docs/source/apis/utils/utils.lazy_import.rst
index 309b4bc4..a443d548 100644
--- a/docs/source/apis/utils/utils.lazy_import.rst
+++ b/docs/source/apis/utils/utils.lazy_import.rst
@@ -1,6 +1,6 @@
.. _utils-lazy_import:
-lazy\_import
+lazy_import
=========================
.. automodule:: utils.lazy_import
diff --git a/docs/source/apis/utils/utils.setup_env.rst b/docs/source/apis/utils/utils.setup_env.rst
index c4a7241a..25c8baa3 100644
--- a/docs/source/apis/utils/utils.setup_env.rst
+++ b/docs/source/apis/utils/utils.setup_env.rst
@@ -1,6 +1,6 @@
.. _utils-setup_env:
-setup\_env
+setup_env
=======================
.. automodule:: utils.setup_env
diff --git a/docs/source/change_api_file_name.py b/docs/source/change_api_file_name.py
index a5e8ea4b..fedaa4f0 100644
--- a/docs/source/change_api_file_name.py
+++ b/docs/source/change_api_file_name.py
@@ -1,29 +1,49 @@
import os
-import re
+
+
+def remove_bom(s):
+ # Remove BOM if present
+ return s.encode().decode("utf-8-sig")
+
def update_file_content(directory: str):
- module_name = directory.split("/")[-1] if "_autosummary" not in directory else "components"
+ module_name = (
+ directory.split("/")[-1] if "_autosummary" not in directory else "components"
+ )
# print(f"directory: {directory}; module_name {module_name}")
for filename in os.listdir(directory):
# print(filename)
if filename.endswith(".rst") and "index" not in filename:
filepath = os.path.join(directory, filename)
- # print(filepath)
- with open(filepath, "r+", encoding='utf-8') as file:
+ print(filepath, module_name)
+ with open(filepath, "r+", encoding="utf-8") as file:
lines = file.readlines()
modified = False # To track if modifications have been made
+ if modified:
+ break
for i in range(len(lines) - 1):
- line = lines[i].strip()
+ line = remove_bom(lines[i].strip())
+ # Replace escaped underscores with actual underscores
+ line = line.replace("\\_", "_")
next_line = lines[i + 1].strip()
-
+
# Check if the next line is a title underline
if next_line == "=" * len(next_line) and not modified:
+ print(f"filepath: {filepath}")
+ print(
+ f"line: {repr(line)}, module_name: {repr(module_name)}, {line.startswith(module_name)}"
+ )
+
# Check if the current line starts with the module_name
if line.startswith(module_name):
+ print(f"here: {line}, ")
# Replace the full module path with only the last segment
- new_title = line.split('.')[-1]
+ new_title = line.split(".")[-1]
# print(f"new_title: {new_title}")
- lines[i] = new_title + '\n' # Update the title line
+ lines[i] = new_title + "\n" # Update the title line
+ print(
+ f"Updated title in {filepath}, lnew_title: {new_title}"
+ )
modified = True # Mark that modification has been made
# No need to break since we are preserving the rest of the content
@@ -32,8 +52,7 @@ def update_file_content(directory: str):
file.seek(0)
file.writelines(lines)
file.truncate() # Ensure the file is cut off at the new end if it's shorter
- print(f"Updated {filepath}")
-
+ print(f"Updated {filepath} ")
if __name__ == "__main__":
@@ -41,6 +60,7 @@ def update_file_content(directory: str):
directories = [
"./source/apis/core",
"./source/apis/components",
+ "./source/apis/components/_autosummary",
"./source/apis/utils",
"./source/apis/eval",
"./source/apis/tracing",
@@ -48,4 +68,4 @@ def update_file_content(directory: str):
# "./source/apis/components/_autosummary",
]
for diretory in directories:
- update_file_content(diretory)
\ No newline at end of file
+ update_file_content(diretory)
diff --git a/docs/source/conf.py b/docs/source/conf.py
index d9d059b1..5e4734f3 100644
--- a/docs/source/conf.py
+++ b/docs/source/conf.py
@@ -1,38 +1,13 @@
-# Configuration file for the Sphinx documentation builder.
-#
-# This file only contains a selection of the most common options. For a full
-# list see the documentation:
-# https://www.sphinx-doc.org/en/master/usage/configuration.html
-
-# -- Path setup --------------------------------------------------------------
-
-# If extensions (or modules to document with autodoc) are in another directory,
-# add these directories to sys.path here. If the directory is relative to the
-# documentation root, use os.path.abspath to make it absolute, like shown here.
-#
import os
import sys
-
sys.path.insert(0, os.path.abspath("../../"))
sys.path.insert(0, os.path.abspath("../../lightrag/lightrag"))
-# # need to insert the paths
-# for dir in os.walk('../../lightrag'):
-# sys.path.insert(0, dir[0])
-# # print(dir[0])
-
-
-# -- Project information -----------------------------------------------------
project = "LightRAG"
copyright = "2024, SylphAI, Inc"
author = "SylphAI, Inc"
-# -- General configuration ---------------------------------------------------
-
-# Add any Sphinx extension module names here, as strings. They can be
-# extensions coming with Sphinx (named 'sphinx.ext.*') or your custom
-# ones.
extensions = [
"sphinx.ext.autodoc",
"sphinx.ext.autosummary",
@@ -48,46 +23,26 @@
"sphinx_copybutton",
"nbsphinx",
"sphinx_search.extension",
- # "myst_nb",
- # "sphinx.builders.changes",
- # 'recommonmark',
- # 'myst_parser'
]
html_show_sphinx = False
-
-# Add any paths that contain templates here, relative to this directory.
templates_path = ["_templates"]
-# List of patterns, relative to source directory, that match files and
-# directories to ignore when looking for source files.
-# This pattern also affects html_static_path and html_extra_path.
exclude_patterns = ["lightrag/tests", "test_*", "../li_test"]
-# exclude_patterns = ['_build', 'Thumbs.db', '.DS_Store', '**.module.rst', '**/tests/*', '**/test_*.py', '*test.rst']
-
-# -- Options for HTML output -------------------------------------------------
-
-# The theme to use for HTML and HTML Help pages. See the documentation for
-# a list of builtin themes.
-
html_theme = "pydata_sphinx_theme"
-
+html_show_sourcelink = False
html_logo = "./_static/images/LightRAG-logo-doc.jpeg"
-# These options are for the sphinx_rtd_theme
html_theme_options = {
"collapse_navigation": False,
- # "sticky_navigation": True, # Ensures the sidebar stays at the top of the page
- "navigation_depth": 8, # Controls how many headers are shown in the sidebar
- # "includehidden": True,
- # "titles_only": False,
+ "navigation_depth": 8,
"icon_links": [
{
"name": "GitHub",
- "url": "https://github.com/SylphAI-Inc/LightRAG", # Replace with your GitHub URL
+ "url": "https://github.com/SylphAI-Inc/LightRAG",
"icon": "fa-brands fa-github",
},
{
@@ -96,43 +51,29 @@
"icon": "fa-brands fa-discord",
},
],
- # "navigation_with_keys": True
}
-# Add any paths that contain custom static files (such as style sheets) here,
-# relative to this directory. They are copied after the builtin static files,
-# so a file named "default.css" will overwrite the builtin "default.css".
-html_static_path = ["_static"] # Only for CSS, JS, images, etc.
+html_static_path = ["_static"]
-# A shorter title for the navigation bar. Default is the same as html_title.
html_short_title = "LightRAG"
-# this will be the logo shown on the browser header
html_favicon = "./_static/images/LightRAG-logo-circle.png"
-# In Sphinx documentation, the configuration option add_module_names in the conf.py file controls
-# whether module names are prefixed before object names in the documentation. This setting is particularly
-# relevant when documenting Python modules and their contents, such as classes, functions, and methods.
-autosummary_generate = True # Tells Sphinx to generate summary pages
-autosummary_imported_members = False # Consider turning this off if not necessary
+autosummary_generate = False
+autosummary_imported_members = False
add_module_names = False
autosectionlabel_prefix_document = True
autodoc_docstring_signature = True
-
autodoc_default_options = {
"members": True,
"undoc-members": True,
"member-order": "bysource",
"show-inheritance": True,
- "private-members": False, # Ensure this is True if you want to document private members
- # "special-members": "__init__", # Document special members like __init__
+ "private-members": False,
"inherited-members": False,
"exclude-members": "__init__",
- # "autosectionlabel_prefix_document": True,
}
def setup(app):
- app.add_css_file(
- "css/custom.css"
- ) # Add custom CSS file to the Sphinx configuration
+ app.add_css_file("css/custom.css")
diff --git a/docs/source/developer_notes/class_hierarchy.rst b/docs/source/developer_notes/class_hierarchy.rst
index 931d6e48..0636019b 100644
--- a/docs/source/developer_notes/class_hierarchy.rst
+++ b/docs/source/developer_notes/class_hierarchy.rst
@@ -1,6 +1,6 @@
Class Hierarchy
=============================
-From the plot of the `LightRAG` library's class hierarchy, we can see the library is well-centered around two base classes: `Component` and `DataClass`, and it maintains a class inheritance hierarchy with no more than two levels.
+From the plot of the `LightRAG` library's class hierarchy, we can see the library is well-centered around two powerful base classes: `Component` and `DataClass`, and it maintains no more than two levels of class hierarchy.
This design philosophy results in a library with bare minimum abstraction, providing developers with maximum customizability.
.. raw:: html
diff --git a/docs/source/remove_files.py b/docs/source/remove_files.py
index 38c362e8..08551a25 100644
--- a/docs/source/remove_files.py
+++ b/docs/source/remove_files.py
@@ -46,12 +46,11 @@ def remove_file(directory: str):
"components.model_client.anthropic_client.rst",
"components.output_parsers.outputs.rst",
"components.model_client.cohere_client.rst",
- "components.retriever.reranker_retriever.rst",
+ "components.retriever.reranker_retriever.rst",
"components.data_process.data_components.rst",
"components.data_process.text_splitter.rst",
"components.memory.memory.rst",
- "components.retriever.postgres_retriever.rst"
-
+ "components.retriever.postgres_retriever.rst",
]
try:
for filename in os.listdir(directory):
diff --git a/lightrag/README.md b/lightrag/README.md
index 67e3bc99..34c22a5e 100644
--- a/lightrag/README.md
+++ b/lightrag/README.md
@@ -1,7 +1,7 @@
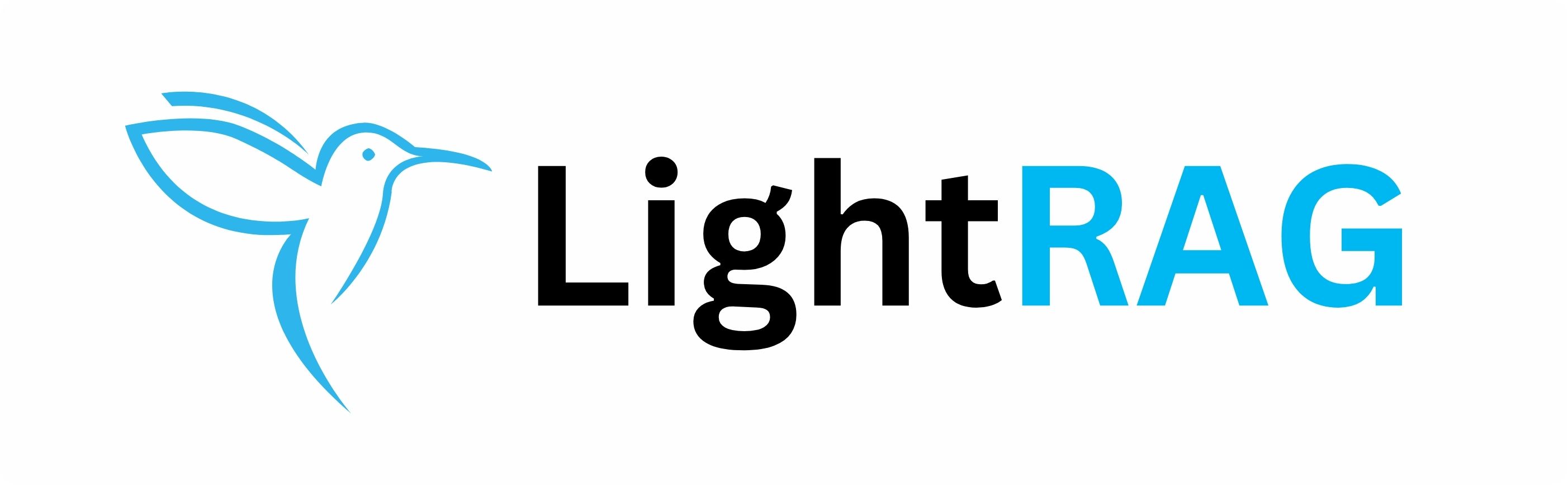
-## ⚡⚡⚡ The PyTorch Library for Large language Model (LLM) Applications ⚡⚡⚡
+### ⚡⚡⚡ The PyTorch Library for Large language Model (LLM) Applications ⚡⚡⚡
*LightRAG* helps developers with both building and optimizing *Retriever-Agent-Generator (RAG)* pipelines.
It is *light*, *modular*, and *robust*.
@@ -82,7 +82,7 @@ LightRAG full documentation available at [lightrag.sylph.ai](https://lightrag.sy
- [Introduction](https://lightrag.sylph.ai/)
- [Full installation guide](https://lightrag.sylph.ai/get_started/installation.html)
- [Design philosophy](https://lightrag.sylph.ai/developer_notes/lightrag_design_philosophy.html): Design based on three principles: Simplicity over complexity, Quality over quantity, and Optimizing over building.
-- [Class hierarchy](https://lightrag.sylph.ai/developer_notes/class_hierarchy.html): We have no more than two levels of subclasses. The bare minimum abstraction will developers with maximum customizability and simplicity.
+- [Class hierarchy](https://lightrag.sylph.ai/developer_notes/class_hierarchy.html): We have no more than two levels of subclasses. The bare minimum abstraction provides developers with maximum customizability and simplicity.
- [Tutorials](https://lightrag.sylph.ai/developer_notes/index.html): Learn the `why` and `how-to` (customize and integrate) behind each core part within the `LightRAG` library.
- [API reference](https://lightrag.sylph.ai/apis/index.html)
@@ -95,11 +95,12 @@ LightRAG full documentation available at [lightrag.sylph.ai](https://lightrag.sy
# Citation
```bibtex
-@software{Yin-LightRAG-2024,
- author = {Yin, Li},
- title = {{LightRAG: The PyTorch Library for Large language Model (LLM) Applications}},
+@software{Yin2024LightRAG,
+ author = {Li Yin},
+ title = {{LightRAG: The PyTorch Library for Large Language Model (LLM) Applications}},
month = {7},
year = {2024},
+ doi = {10.5281/zenodo.12639531},
url = {https://github.com/SylphAI-Inc/LightRAG}
}
```
diff --git a/lightrag/lightrag/components/output_parsers/outputs.py b/lightrag/lightrag/components/output_parsers/outputs.py
index af5b4f51..da6e583b 100644
--- a/lightrag/lightrag/components/output_parsers/outputs.py
+++ b/lightrag/lightrag/components/output_parsers/outputs.py
@@ -171,17 +171,19 @@ def format_instructions(
# convert example to string, convert data class to yaml string
example_str = ""
try:
- for example in self.examples:
- per_example_str = example.format_example_str(
- format_type=DataClassFormatType.EXAMPLE_YAML,
- exclude=self._exclude_fields,
- )
- example_str += f"{per_example_str}\n________\n"
- # remove the last new line
- example_str = example_str[:-1]
- log.debug(f"{__class__.__name__} example_str: {example_str}")
-
- except Exception:
+ if self.examples and len(self.examples) > 0:
+ for example in self.examples:
+ per_example_str = example.format_example_str(
+ format_type=DataClassFormatType.EXAMPLE_YAML,
+ exclude=self._exclude_fields,
+ )
+ example_str += f"{per_example_str}\n________\n"
+ # remove the last new line
+ example_str = example_str[:-1]
+ log.debug(f"{__class__.__name__} example_str: {example_str}")
+
+ except Exception as e:
+ log.error(f"Error in formatting example for {__class__.__name__}, {e}")
example_str = None
return self.yaml_output_format_prompt(schema=schema, example=example_str)
@@ -240,18 +242,19 @@ def format_instructions(
)
example_str = ""
try:
- for example in self.examples:
- per_example_str = example.format_example_str(
- format_type=DataClassFormatType.EXAMPLE_JSON,
- exclude=self._exclude_fields,
- )
- example_str += f"{per_example_str}\n________\n"
- # remove the last new line
- example_str = example_str[:-1]
- log.debug(f"{__class__.__name__} example_str: {example_str}")
-
- except Exception:
- log.error(f"Error in formatting example for {__class__.__name__}")
+ if self.examples and len(self.examples) > 0:
+ for example in self.examples:
+ per_example_str = example.format_example_str(
+ format_type=DataClassFormatType.EXAMPLE_JSON,
+ exclude=self._exclude_fields,
+ )
+ example_str += f"{per_example_str}\n________\n"
+ # remove the last new line
+ example_str = example_str[:-1]
+ log.debug(f"{__class__.__name__} example_str: {example_str}")
+
+ except Exception as e:
+ log.error(f"Error in formatting example for {__class__.__name__}, {e}")
example_str = None
return self.json_output_format_prompt(schema=schema, example=example_str)
diff --git a/lightrag/pyproject.toml b/lightrag/pyproject.toml
index 81e530b1..6226b0e4 100644
--- a/lightrag/pyproject.toml
+++ b/lightrag/pyproject.toml
@@ -1,7 +1,7 @@
[tool.poetry]
name = "lightrag"
-version = "0.0.0-alpha.9"
+version = "0.0.0-alpha.11"
description = "The 'PyTorch' library for LLM applications. RAG=Retriever-Agent-Generator."
authors = ["Li Yin "]
readme = "README.md"
diff --git a/lightrag/tests/_test_openai_client.py b/lightrag/tests/_test_openai_client.py
index 5754d978..10725aba 100644
--- a/lightrag/tests/_test_openai_client.py
+++ b/lightrag/tests/_test_openai_client.py
@@ -1,8 +1,8 @@
import unittest
from unittest.mock import patch, AsyncMock
-from lightrag.core.base_data_class import ModelType
-from lightrag.components.api_client import OpenAIClient
+from lightrag.core.types import ModelType
+from lightrag.components.model_client import OpenAIClient
def getenv_side_effect(key):
diff --git a/test_pyvis.py b/test_pyvis.py
deleted file mode 100644
index 39f72639..00000000
--- a/test_pyvis.py
+++ /dev/null
@@ -1,15 +0,0 @@
-from pyvis.network import Network
-import networkx as nx
-
-# Create a simple NetworkX graph
-G = nx.DiGraph()
-G.add_edges_from([(1, 2), (2, 3), (3, 4)])
-
-# Create a Pyvis Network
-net = Network(notebook=False, width="100%", height="100%", directed=True)
-
-# Add nodes and edges from NetworkX to Pyvis
-net.from_nx(G)
-
-# Save the network to an HTML file
-net.show("test_network.html")
diff --git a/use_cases/simple_qa_anthropic.py b/use_cases/simple_qa_anthropic.py
index 63273354..0c2dc8a2 100644
--- a/use_cases/simple_qa_anthropic.py
+++ b/use_cases/simple_qa_anthropic.py
@@ -14,7 +14,7 @@ def __init__(self):
self.generator = Generator(
model_client=AnthropicAPIClient(),
model_kwargs={"model": "claude-3-opus-20240229", "max_tokens": 1000},
- preset_prompt_kwargs={
+ prompt_kwargs={
"task_desc_str": "You are a helpful assistant and with a great sense of humor."
},
)
diff --git a/use_cases/tracing/trace_generator.py b/use_cases/tracing/trace_generator.py
index d4079658..98bab43f 100644
--- a/use_cases/tracing/trace_generator.py
+++ b/use_cases/tracing/trace_generator.py
@@ -1,10 +1,8 @@
-from core.generator import Generator
-from core.component import Component
+from lightrag.core.generator import Generator
+from lightrag.core.component import Component
-from components.api_client import GroqAPIClient
-from tracing.decorators import trace_generator_states, trace_generator_call
-
-import utils.setup_env
+from lightrag.components.model_client import GroqAPIClient
+from lightrag.tracing.decorators import trace_generator_states, trace_generator_call
@trace_generator_states()
@@ -35,7 +33,7 @@ def call(self, query: str) -> str:
if __name__ == "__main__":
- from utils import enable_library_logging, get_logger
+ from lightrag.utils import enable_library_logging, get_logger
enable_library_logging(enable_file=False, level="DEBUG")
log = get_logger(__name__, level="INFO")
diff --git a/visualize.py b/visualize.py
index 971d6e65..cf865641 100644
--- a/visualize.py
+++ b/visualize.py
@@ -163,7 +163,7 @@ def process_directory(directory):
# Directory containing your Python files
def light_rag_paths():
- Light_rag_directory = "/Users/liyin/Documents/test/LightRAG/lightrag"
+ Light_rag_directory = "/Users/liyin/Documents/test/LightRAG/lightrag/lightrag"
paths = ["core", "components", "utils", "eval", "optim", "tracing"]
for path in paths: