diff --git a/.pre-commit-config.yaml b/.pre-commit-config.yaml index a2cd1504..587f23bd 100644 --- a/.pre-commit-config.yaml +++ b/.pre-commit-config.yaml @@ -33,6 +33,7 @@ repos: .*node_modules.*| .*boilerplate.*| .*src.*.js| + builder/public/js/identify.js| )$ ci: diff --git a/README.md b/README.md index 87810b92..706a0e42 100644 --- a/README.md +++ b/README.md @@ -5,7 +5,7 @@
Crafting Web Pages Made Effortless!
-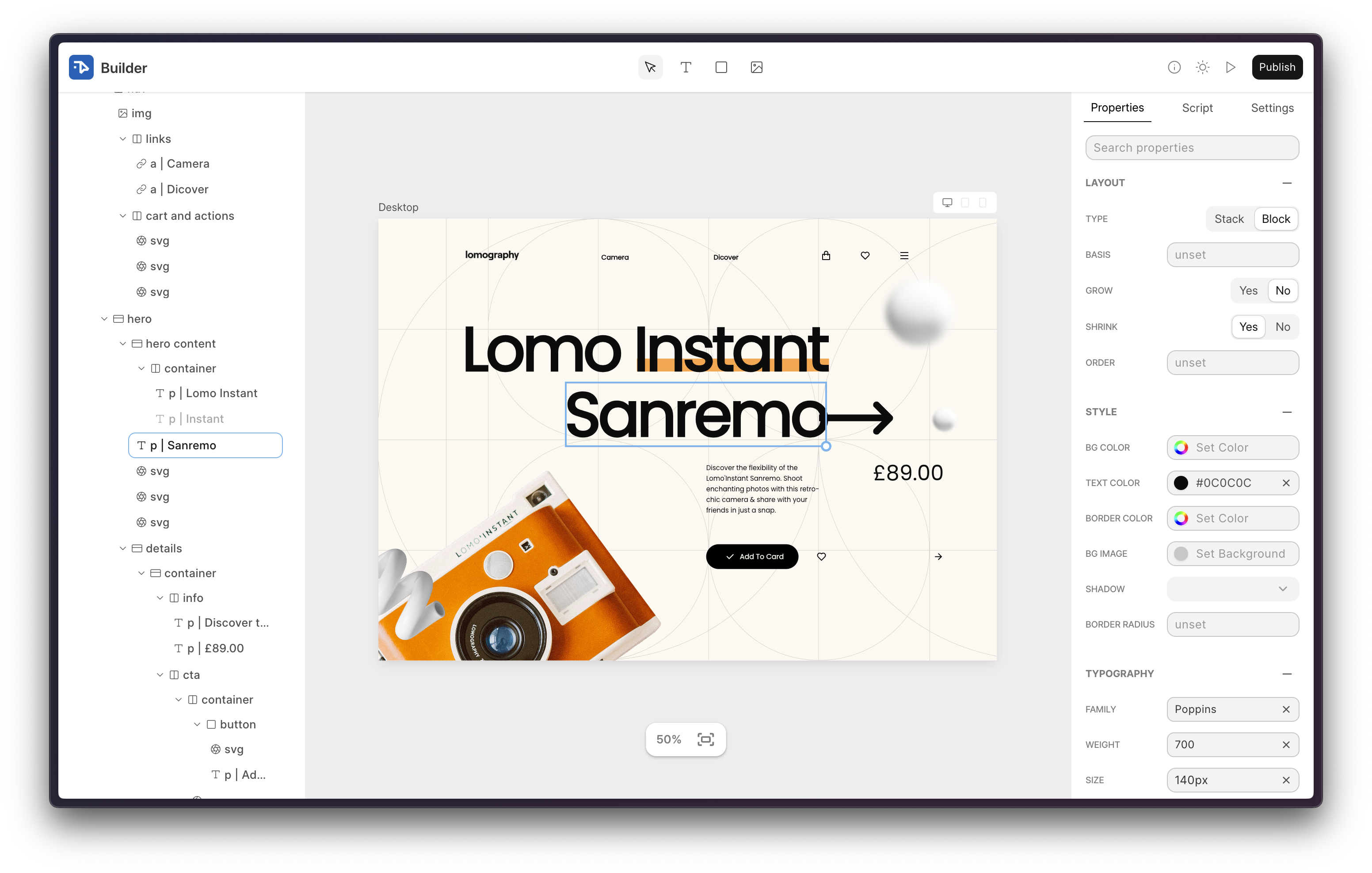 + [Web page design credit](https://www.figma.com/community/file/949266436474872912) diff --git a/builder/api.py b/builder/api.py index 8a781691..4f9f01e8 100644 --- a/builder/api.py +++ b/builder/api.py @@ -1,8 +1,20 @@ import json +import os +from io import BytesIO +from urllib.parse import unquote import frappe +import requests +from frappe.apps import get_apps as get_permitted_apps +from frappe.core.doctype.file.file import get_local_image +from frappe.core.doctype.file.utils import delete_file from frappe.integrations.utils import make_post_request +from frappe.model.document import Document +from frappe.utils.caching import redis_cache from frappe.utils.telemetry import POSTHOG_HOST_FIELD, POSTHOG_PROJECT_FIELD +from PIL import Image + +from builder.builder.doctype.builder_page.builder_page import BuilderPageRenderer @frappe.whitelist() @@ -52,6 +64,132 @@ def get_posthog_settings(): } +@frappe.whitelist() +def get_page_preview_html(page: str, **kwarg) -> str: + # to load preview without publishing + frappe.form_dict.update(kwarg) + renderer = BuilderPageRenderer(path="") + renderer.docname = page + renderer.doctype = "Builder Page" + frappe.flags.show_preview = True + frappe.local.no_cache = 1 + renderer.init_context() + response = renderer.render() + page = frappe.get_cached_doc("Builder Page", page) + frappe.enqueue_doc( + page.doctype, + page.name, + "generate_page_preview_image", + html=str(response.data, "utf-8"), + queue="short", + ) + return response + + +@frappe.whitelist() +def upload_builder_asset(): + from frappe.handler import upload_file + + image_file = upload_file() + if image_file.file_url.endswith((".png", ".jpeg", ".jpg")) and frappe.get_cached_value( + "Builder Settings", None, "auto_convert_images_to_webp" + ): + convert_to_webp(file_doc=image_file) + return image_file + + +@frappe.whitelist() +def convert_to_webp(image_url: str | None = None, file_doc: Document | None = None) -> str: + """BETA: Convert image to webp format""" + + CONVERTIBLE_IMAGE_EXTENSIONS = ["png", "jpeg", "jpg"] + + def can_convert_image(extn): + return extn.lower() in CONVERTIBLE_IMAGE_EXTENSIONS + + def get_extension(filename): + return filename.split(".")[-1].lower() + + def convert_and_save_image(image, path): + image.save(path, "WEBP") + return path + + def update_file_doc_with_webp(file_doc, image, extn): + webp_path = file_doc.get_full_path().replace(extn, "webp") + convert_and_save_image(image, webp_path) + delete_file(file_doc.get_full_path()) + file_doc.file_url = f"{file_doc.file_url.replace(extn, 'webp')}" + file_doc.save() + return file_doc.file_url + + def create_new_webp_file_doc(file_url, image, extn): + files = frappe.get_all("File", filters={"file_url": file_url}, fields=["name"], limit=1) + if files: + _file = frappe.get_doc("File", files[0].name) + webp_path = _file.get_full_path().replace(extn, "webp") + convert_and_save_image(image, webp_path) + new_file = frappe.copy_doc(_file) + new_file.file_name = f"{_file.file_name.replace(extn, 'webp')}" + new_file.file_url = f"{_file.file_url.replace(extn, 'webp')}" + new_file.save() + return new_file.file_url + return file_url + + def handle_image_from_url(image_url): + image_url = unquote(image_url) + response = requests.get(image_url) + image = Image.open(BytesIO(response.content)) + filename = image_url.split("/")[-1] + extn = get_extension(filename) + if can_convert_image(extn): + _file = frappe.get_doc( + { + "doctype": "File", + "file_name": f"{filename.replace(extn, 'webp')}", + "file_url": f"/files/{filename.replace(extn, 'webp')}", + } + ) + webp_path = _file.get_full_path() + convert_and_save_image(image, webp_path) + _file.save() + return _file.file_url + return image_url + + if not image_url and not file_doc: + return "" + + if file_doc: + if file_doc.file_url.startswith("/files"): + image, filename, extn = get_local_image(file_doc.file_url) + if can_convert_image(extn): + return update_file_doc_with_webp(file_doc, image, extn) + return file_doc.file_url + + if image_url.startswith("/files"): + image, filename, extn = get_local_image(image_url) + if can_convert_image(extn): + return create_new_webp_file_doc(image_url, image, extn) + return image_url + + if image_url.startswith("/builder_assets"): + image_path = os.path.abspath(frappe.get_app_path("builder", "www", image_url.lstrip("/"))) + image_path = image_path.replace("_", "-") + image_path = image_path.replace("/builder-assets", "/builder_assets") + + image = Image.open(image_path) + extn = get_extension(image_path) + if can_convert_image(extn): + webp_path = image_path.replace(extn, "webp") + convert_and_save_image(image, webp_path) + return image_url.replace(extn, "webp") + return image_url + + if image_url.startswith("http"): + return handle_image_from_url(image_url) + + return image_url + + def check_app_permission(): if frappe.session.user == "Administrator": return True @@ -60,3 +198,20 @@ def check_app_permission(): return True return False + + +@frappe.whitelist() +@redis_cache() +def get_apps(): + apps = get_permitted_apps() + app_list = [ + { + "name": "frappe", + "logo": "/assets/builder/images/desk.png", + "title": "Desk", + "route": "/app", + } + ] + app_list += filter(lambda app: app.get("name") != "builder", apps) + + return app_list diff --git a/builder/builder/builder_block_template/blockquote/blockquote.json b/builder/builder/builder_block_template/blockquote/blockquote.json new file mode 100644 index 00000000..e58a3b1d --- /dev/null +++ b/builder/builder/builder_block_template/blockquote/blockquote.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"left\": \"auto\",\"overflow\": \"hidden\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"100%\"},\"blockId\": \"udqa5zyxm\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"position\": \"static\",\"width\": \"fit-content\"},\"blockId\": \"98f44tdju\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Words can be like X-rays, if you use them properly\\u2014they\\u2019ll go through anything. You read and you\\u2019re pierced.
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"6px\",\"height\": \"fit-content\",\"overflow\": \"hidden\",\"position\": \"relative\",\"width\": \"fit-content\"},\"blockId\": \"ztvga4ewc\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"height\": \"fit-content\",\"width\": \"fit-content\"},\"blockId\": \"pxv3ojq7t\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\\u2014Aldous Huxley,\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"background\": \"#e2e2e2\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"overflow\": \"hidden\",\"position\": \"static\"},\"blockId\": \"bglu90k42\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"cite\",\"innerHTML\": \"
Brave New World
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {\"cite\": \"https://www.huxley.net/bnw/four.html\"},\"dataKey\": null,\"element\": \"blockquote\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Typography", + "creation": "2024-09-03 23:17:52.830414", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:10:00.947270", + "modified_by": "Administrator", + "name": "Blockquote", + "order": 13, + "owner": "Administrator", + "preview": "/builder_assets/Blockquote/blockquote.png", + "preview_height": 1, + "preview_width": 1, + "template_name": "Blockquote" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/button/button.json b/builder/builder/builder_block_template/button/button.json index 83704ce1..ec9d662a 100644 --- a/builder/builder/builder_block_template/button/button.json +++ b/builder/builder/builder_block_template/button/button.json @@ -1,14 +1,17 @@ { - "block": "{\"blockId\":\"4ur2l2mc6\",\"children\":[{\"blockId\":\"3z5gwjl2f\",\"children\":[],\"baseStyles\":{\"color\":\"var(--neutral-white, #FFF)\",\"fontSize\":\"14px\",\"fontWeight\":\"420\",\"height\":\"fit-content\",\"left\":\"auto\",\"letterSpacing\":\"0.28px\",\"lineHeight\":\"115%\",\"minWidth\":\"30px\",\"position\":\"static\",\"top\":\"auto\",\"width\":\"fit-content\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[\"__text_block__\"],\"dataKey\":null,\"element\":\"p\",\"innerHTML\":\"Button
\",\"customAttributes\":{}}],\"baseStyles\":{\"background\":\"#171717\",\"borderRadius\":\"4px\",\"display\":\"flex\",\"flexDirection\":\"column\",\"height\":\"fit-content\",\"padding\":\"6px 8px\",\"width\":\"fit-content\"},\"rawStyles\":{\"flex-shrink\":\"0\",\"hover:background\":\"#383838\",\"transition\":\"all 0.1s ease-out\"},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"href\":\"#\"},\"classes\":[\"__text_block__\"],\"dataKey\":null,\"blockName\":\"container\",\"element\":\"a\",\"customAttributes\":{}}", + "block": "{\"attributes\": {\"href\": \"#\"},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#171717\",\"borderRadius\": \"4px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"height\": \"fit-content\",\"justifyContent\": \"center\",\"padding\": \"6px 8px\",\"width\": \"fit-content\"},\"blockId\": \"4ur2l2mc6\",\"blockName\": \"button-link\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"var(--neutral-white, #FFF)\",\"fontSize\": \"14px\",\"fontWeight\": \"420\",\"height\": \"fit-content\",\"left\": \"auto\",\"letterSpacing\": \"0.28px\",\"lineHeight\": \"115%\",\"minWidth\": \"30px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"fit-content\"},\"blockId\": \"3z5gwjl2f\",\"children\": [],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Button
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"editable\": false,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {\"flex-shrink\": \"0\",\"hover:background\": \"#383838\",\"transition\": \"all 0.1s ease-out\"},\"tabletStyles\": {}}", "category": "Basic", "creation": "2024-07-10 14:44:08.816465", "docstatus": 0, "doctype": "Block Template", "idx": 0, - "modified": "2024-07-12 09:34:22.716694", + "modified": "2024-09-19 13:08:10.275886", "modified_by": "Administrator", "name": "Button", + "order": 1, "owner": "Administrator", "preview": "/builder_assets/Button/button.png", + "preview_height": 1, + "preview_width": 1, "template_name": "Button" } \ No newline at end of file diff --git a/builder/builder/builder_block_template/embed/embed.json b/builder/builder/builder_block_template/embed/embed.json index 73a3f651..ea5a0b6a 100644 --- a/builder/builder/builder_block_template/embed/embed.json +++ b/builder/builder/builder_block_template/embed/embed.json @@ -1,11 +1,11 @@ { "block": "{\"blockId\":\"1vw78v0md\",\"children\":[],\"baseStyles\":{\"height\":\"fit-content\",\"width\":\"fit-content\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[],\"dataKey\":null,\"blockName\":\"Embed HTML\",\"element\":\"div\",\"innerHTML\":\"Paste HTML or double click to edit
\\nTransform your designs into stunning websites with Frappe builder, the ultimate web builder for creative pros.
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-end\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"23px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"10px\",\"paddingRight\": \"10px\",\"paddingTop\": \"10px\"},\"blockId\": \"1otqfemvd\",\"blockName\": \"social\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"xdjj348b7\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {},\"blockId\": \"u2xkwijlw\",\"blockName\": \"facebook\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"svg\",\"innerHTML\": \"\\n\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"8o6mm2cdb\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {},\"blockId\": \"u1ekxhiv1\",\"blockName\": \"twitter\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"svg\",\"innerHTML\": \"\\n\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"r4iv0wuoc\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {},\"blockId\": \"r3fudne7b\",\"blockName\": \"medium\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"svg\",\"innerHTML\": \"\\n\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"pyba60bv5\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {},\"blockId\": \"tagf2z51x\",\"blockName\": \"github\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"svg\",\"innerHTML\": \"\\n\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"width\": \"100%\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {\"gap\": \"20px\",\"width\": \"273px\"}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"10px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"nqbjj11d7\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"20px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\",\"width\": \"200px\"},\"blockId\": \"d4w69s8eg\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"8px\",\"height\": \"24px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"07jtmm84h\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(23, 23, 23)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"-0.5%\",\"lineHeight\": \"150%\"},\"blockId\": \"zcyjuogrf\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Services
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"12px\",\"height\": \"132px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"z4n1d2i83\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"ktqrphv2q\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Cloud
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"skfy075b8\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Support\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"si8qyc9s1\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Mobile apps\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"ygnijnps6\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Login
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"width\": \"45%\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {\"width\": \"100px\"}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"20px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\",\"width\": \"200px\"},\"blockId\": \"4n8qmplt8\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"8px\",\"height\": \"24px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"yp0fqrqdd\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(23, 23, 23)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"-0.5%\",\"lineHeight\": \"150%\"},\"blockId\": \"105alltts\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Apps
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"12px\",\"height\": \"168px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"kdq1dow5i\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"doh9t5a12\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Gameplan\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"6p08a3pse\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Insights
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"ycynx3igy\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"CRM
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"f3ue51wtf\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Builder\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"iu3pg6g3j\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"See more
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"width\": \"45%\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {\"width\": \"100px\"}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"20px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\",\"width\": \"200px\"},\"blockId\": \"cfjzm6god\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"8px\",\"height\": \"24px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"essjxuapl\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(23, 23, 23)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"-0.5%\",\"lineHeight\": \"150%\"},\"blockId\": \"9v1rofnpa\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Company
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"12px\",\"height\": \"168px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"7hg3h8por\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"wey03z8ha\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"About
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"sn82zbglu\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Blog
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"wvl2u43uq\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Products\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"cnwzbff05\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Features\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"xa5nc7fc1\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Pricing\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"width\": \"45%\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {\"width\": \"100px\"}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"20px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\",\"width\": \"200px\"},\"blockId\": \"53990huoy\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"8px\",\"height\": \"24px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"3vussng0p\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(23, 23, 23)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"-0.5%\",\"lineHeight\": \"150%\"},\"blockId\": \"q6int684n\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"What\\u2019s new
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"flex-start\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"12px\",\"height\": \"132px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"vafhal75z\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"8a8oms3cb\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Version 1.1.1
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"sipclbpdw\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Version 1.2.1\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"025ltoff9\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Version 1.3.1\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"16px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"6cvbizhgo\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Version 1.6.1
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"width\": \"45%\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {\"width\": \"100px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"flexWrap\": \"wrap\",\"gap\": \"10px\"},\"originalElement\": \"\",\"rawStyles\": {\"order\": \"1\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"flexDirection\": \"column\",\"gap\": \"50px\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"justifyContent\": \"space-between\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\",\"width\": \"100%\"},\"blockId\": \"0kwur41vl\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"8px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"10px\",\"paddingRight\": \"10px\",\"paddingTop\": \"0px\"},\"blockId\": \"y8gk3bnj1\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(124, 124, 124)\",\"display\": \"flex\",\"fontSize\": \"14px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\"},\"blockId\": \"e1s8u3buh\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"\\u00a9 2024 Your Company, Inc. All rights reserved.
\",\"mobileStyles\": {\"width\": \"100%\"},\"originalElement\": \"\",\"rawStyles\": {\"whiteSpace\": \"wrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {\"paddingLeft\": \"0px\",\"width\": \"100%\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"10px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"fui1hqr7b\",\"children\": [{\"attributes\": {\"placeholder\": \"Enter your email\"},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"rgb(243, 243, 243)\",\"borderRadius\": \"8px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"14px\",\"height\": \"28px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"6px\",\"paddingLeft\": \"8px\",\"paddingRight\": \"8px\",\"paddingTop\": \"6px\",\"width\": \"200px\"},\"blockId\": \"uilntcr4m\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"input\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"boxShadow\": \"0px 0px 0px rgb(199, 199, 199)\"},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"rgb(23, 23, 23)\",\"borderRadius\": \"8px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"8px\",\"justifyContent\": \"center\",\"paddingBottom\": \"6px\",\"paddingLeft\": \"8px\",\"paddingRight\": \"8px\",\"paddingTop\": \"6px\"},\"blockId\": \"ipp7b45nb\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(255, 255, 255)\",\"display\": \"flex\",\"fontSize\": \"14px\",\"letterSpacing\": \"0.5%\",\"lineHeight\": \"114.99999761581421%\"},\"blockId\": \"ivmdmqxka\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Subscribe
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {\"overflow\": \"hidden\",\"textOverflow\": \"ellipsis\",\"whiteSpace\": \"nowrap\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"button\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"width\": \"100%\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"flexDirection\": \"column\",\"gap\": \"20px\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"gap\": \"67px\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"footer\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Structure", + "creation": "2024-09-18 19:52:15.072947", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-10-14 00:07:01.491709", + "modified_by": "Administrator", + "name": "Footer 1", + "order": 7, + "owner": "Administrator", + "preview": "/builder_assets/Footer 1/footer_1.png", + "preview_height": 2, + "preview_width": 2, + "template_name": "Footer 1" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/form_1/form_1.json b/builder/builder/builder_block_template/form_1/form_1.json index c9cb9ac5..9a0ece40 100644 --- a/builder/builder/builder_block_template/form_1/form_1.json +++ b/builder/builder/builder_block_template/form_1/form_1.json @@ -1,14 +1,17 @@ { - "block": "{\"blockId\":\"2e45tti0c\",\"children\":[{\"blockId\":\"86oxik4s9\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"width\":\"100%\",\"height\":\"32px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"Full Name\"},\"classes\":[],\"dataKey\":null,\"element\":\"input\",\"customAttributes\":{\"name\":\"full_name\",\"required\":\"true\"}},{\"blockId\":\"wzbvukqlo\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"width\":\"100%\",\"height\":\"32px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"Email\"},\"classes\":[],\"dataKey\":null,\"element\":\"input\",\"customAttributes\":{\"name\":\"email\",\"required\":\"true\"}},{\"blockId\":\"4rclutff4\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"top\":\"auto\",\"left\":\"auto\",\"width\":\"100%\",\"height\":\"150px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"Message\"},\"classes\":[],\"dataKey\":null,\"element\":\"textarea\",\"customAttributes\":{\"name\":\"message\",\"required\":\"true\"}},{\"blockId\":\"uxry21e1i\",\"children\":[{\"blockId\":\"yjglepmnh\",\"children\":[],\"baseStyles\":{\"color\":\"var(--neutral-white, #FFF)\",\"fontSize\":\"14px\",\"fontWeight\":\"420\",\"height\":\"fit-content\",\"left\":\"auto\",\"letterSpacing\":\"0.28px\",\"lineHeight\":\"115%\",\"minWidth\":\"30px\",\"position\":\"static\",\"top\":\"auto\",\"width\":\"fit-content\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[\"__text_block__\"],\"dataKey\":null,\"element\":\"p\",\"innerHTML\":\"Submit
\",\"customAttributes\":{}}],\"baseStyles\":{\"background\":\"#171717\",\"borderRadius\":\"4px\",\"display\":\"flex\",\"flexDirection\":\"column\",\"height\":\"fit-content\",\"padding\":\"6px 8px\",\"width\":\"100%\",\"justifyContent\":\"flex-start\",\"alignItems\":\"center\"},\"rawStyles\":{\"flex-shrink\":\"0\",\"hover:background\":\"#383838\",\"transition\":\"all 0.1s ease-out\"},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[\"\"],\"dataKey\":null,\"element\":\"button\",\"customAttributes\":{}}],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"top\":\"auto\",\"left\":\"auto\",\"width\":\"100%\",\"paddingLeft\":\"20px\",\"paddingRight\":\"20px\",\"paddingBottom\":\"20px\",\"paddingTop\":\"20px\",\"maxWidth\":\"400px\",\"gap\":\"15px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[],\"dataKey\":null,\"element\":\"form\",\"customAttributes\":{}}", - "category": "Basic", + "block": "{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"gap\": \"15px\",\"left\": \"auto\",\"maxWidth\": \"400px\",\"overflow\": \"hidden\",\"paddingBottom\": \"20px\",\"paddingLeft\": \"20px\",\"paddingRight\": \"20px\",\"paddingTop\": \"20px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"100%\"},\"blockId\": \"2e45tti0c\",\"children\": [{\"attributes\": {\"placeholder\": \"Full Name\"},\"baseStyles\": {\"borderColor\": \"#c9c9c9\",\"borderRadius\": \"4px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"fontSize\": \"14px\",\"height\": \"32px\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"86oxik4s9\",\"children\": [],\"classes\": [],\"customAttributes\": {\"name\": \"full_name\",\"required\": \"true\"},\"dataKey\": null,\"element\": \"input\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {\"placeholder\": \"Email\"},\"baseStyles\": {\"borderColor\": \"#c9c9c9\",\"borderRadius\": \"4px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"fontSize\": \"14px\",\"height\": \"32px\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"wzbvukqlo\",\"children\": [],\"classes\": [],\"customAttributes\": {\"name\": \"email_id\",\"required\": \"true\"},\"dataKey\": null,\"element\": \"input\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {\"placeholder\": \"Message\"},\"baseStyles\": {\"borderColor\": \"#c9c9c9\",\"borderRadius\": \"4px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"fontSize\": \"14px\",\"height\": \"150px\",\"left\": \"auto\",\"overflow\": \"hidden\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"100%\"},\"blockId\": \"4rclutff4\",\"children\": [],\"classes\": [],\"customAttributes\": {\"name\": \"message\",\"required\": \"true\"},\"dataKey\": null,\"element\": \"textarea\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#171717\",\"borderRadius\": \"4px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"height\": \"fit-content\",\"justifyContent\": \"flex-start\",\"padding\": \"6px 8px\",\"width\": \"100%\"},\"blockId\": \"uxry21e1i\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"var(--neutral-white, #FFF)\",\"fontSize\": \"14px\",\"fontWeight\": \"420\",\"height\": \"fit-content\",\"left\": \"auto\",\"letterSpacing\": \"0.28px\",\"lineHeight\": \"115%\",\"minWidth\": \"30px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"fit-content\"},\"blockId\": \"yjglepmnh\",\"children\": [],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Submit
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [\"\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"button\",\"mobileStyles\": {},\"rawStyles\": {\"flex-shrink\": \"0\",\"hover:background\": \"#383838\",\"transition\": \"all 0.1s ease-out\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"form\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Basic Forms", "creation": "2024-07-11 23:34:22.558908", "docstatus": 0, "doctype": "Block Template", "idx": 0, - "modified": "2024-08-05 10:09:12.451684", + "modified": "2024-09-19 13:10:03.603174", "modified_by": "Administrator", "name": "Form 1", + "order": 14, "owner": "Administrator", "preview": "/builder_assets/Form 1/form-1.png", + "preview_height": 1, + "preview_width": 1, "template_name": "Form 1" } \ No newline at end of file diff --git a/builder/builder/builder_block_template/form_2/form_2.json b/builder/builder/builder_block_template/form_2/form_2.json index a39649ee..7b83c52f 100644 --- a/builder/builder/builder_block_template/form_2/form_2.json +++ b/builder/builder/builder_block_template/form_2/form_2.json @@ -1,14 +1,17 @@ { - "block": "{\"blockId\":\"6um6dfg30\",\"children\":[{\"blockId\":\"kv3hkfwvl\",\"children\":[{\"blockId\":\"a6gbqj3zl\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":1,\"overflow\":\"hidden\",\"position\":\"static\",\"width\":\"100%\",\"height\":\"28px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{\"border-right\":\"none\",\"border-top-right-radius\":\"0px\",\"border-bottom-right-radius\":\"0px\"},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"Email\",\"type\":\"email\"},\"classes\":[],\"dataKey\":null,\"element\":\"input\",\"customAttributes\":{\"name\":\"email\",\"required\":\"true\"}}],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"row\",\"flexShrink\":1,\"height\":\"fit-content\",\"width\":\"100%\",\"overflow\":\"hidden\",\"position\":\"relative\",\"gap\":\"10px\",\"flexGrow\":0},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[],\"dataKey\":null,\"blockName\":\"container\",\"element\":\"div\",\"customAttributes\":{}},{\"blockId\":\"m68wwxf5e\",\"children\":[{\"blockId\":\"0aw9cg9y7\",\"children\":[],\"baseStyles\":{\"color\":\"var(--neutral-white, #FFF)\",\"fontSize\":\"14px\",\"fontWeight\":\"420\",\"height\":\"fit-content\",\"left\":\"auto\",\"letterSpacing\":\"0.28px\",\"lineHeight\":\"115%\",\"minWidth\":\"30px\",\"position\":\"static\",\"top\":\"auto\",\"width\":\"fit-content\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[\"__text_block__\"],\"dataKey\":null,\"element\":\"p\",\"innerHTML\":\"Sign Up
\",\"customAttributes\":{}}],\"baseStyles\":{\"background\":\"#171717\",\"borderRadius\":\"4px\",\"display\":\"flex\",\"flexDirection\":\"column\",\"height\":\"fit-content\",\"padding\":\"6px 8px\",\"justifyContent\":\"flex-start\",\"alignItems\":\"center\"},\"rawStyles\":{\"flex-shrink\":\"0\",\"hover:background\":\"#383838\",\"transition\":\"all 0.1s ease-out\",\"border-top-left-radius\":\"0px\",\"border-bottom-left-radius\":\"0px\"},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[\"\"],\"dataKey\":null,\"element\":\"button\",\"customAttributes\":{}}],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"row\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"top\":\"auto\",\"left\":\"auto\",\"width\":\"100%\",\"paddingLeft\":\"20px\",\"paddingRight\":\"20px\",\"paddingBottom\":\"20px\",\"paddingTop\":\"20px\",\"maxWidth\":\"400px\",\"gap\":\"0px\",\"justifyContent\":\"flex-start\",\"alignItems\":\"center\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[],\"dataKey\":null,\"element\":\"form\",\"customAttributes\":{}}", - "category": "Basic", + "block": "{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"0px\",\"justifyContent\": \"flex-start\",\"left\": \"auto\",\"maxWidth\": \"400px\",\"overflow\": \"hidden\",\"paddingBottom\": \"20px\",\"paddingLeft\": \"20px\",\"paddingRight\": \"20px\",\"paddingTop\": \"20px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"100%\"},\"blockId\": \"6um6dfg30\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"row\",\"flexGrow\": 0,\"flexShrink\": 1,\"gap\": \"10px\",\"height\": \"fit-content\",\"overflow\": \"hidden\",\"position\": \"relative\",\"width\": \"100%\"},\"blockId\": \"kv3hkfwvl\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"placeholder\": \"Email\",\"type\": \"email\"},\"baseStyles\": {\"borderColor\": \"#c9c9c9\",\"borderRadius\": \"4px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 1,\"fontSize\": \"14px\",\"height\": \"28px\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"a6gbqj3zl\",\"children\": [],\"classes\": [],\"customAttributes\": {\"name\": \"email\",\"required\": \"true\"},\"dataKey\": null,\"element\": \"input\",\"mobileStyles\": {},\"rawStyles\": {\"border-bottom-right-radius\": \"0px\",\"border-right\": \"none\",\"border-top-right-radius\": \"0px\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#171717\",\"borderRadius\": \"4px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"height\": \"fit-content\",\"justifyContent\": \"flex-start\",\"padding\": \"6px 8px\"},\"blockId\": \"m68wwxf5e\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"var(--neutral-white, #FFF)\",\"fontSize\": \"14px\",\"fontWeight\": \"420\",\"height\": \"fit-content\",\"left\": \"auto\",\"letterSpacing\": \"0.28px\",\"lineHeight\": \"115%\",\"minWidth\": \"30px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"fit-content\"},\"blockId\": \"0aw9cg9y7\",\"children\": [],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Sign Up
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [\"\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"button\",\"mobileStyles\": {},\"rawStyles\": {\"border-bottom-left-radius\": \"0px\",\"border-top-left-radius\": \"0px\",\"flex-shrink\": \"0\",\"hover:background\": \"#383838\",\"transition\": \"all 0.1s ease-out\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"form\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Basic Forms", "creation": "2024-07-12 00:27:46.067242", "docstatus": 0, "doctype": "Block Template", "idx": 0, - "modified": "2024-08-05 10:08:57.335197", + "modified": "2024-09-19 13:10:05.562456", "modified_by": "Administrator", "name": "Form 2", + "order": 15, "owner": "Administrator", "preview": "/builder_assets/Form 2/form-2.png", + "preview_height": 1, + "preview_width": 1, "template_name": "Form 2" } \ No newline at end of file diff --git a/builder/builder/builder_block_template/form_3/form_3.json b/builder/builder/builder_block_template/form_3/form_3.json index 9dc4caf3..e0455699 100644 --- a/builder/builder/builder_block_template/form_3/form_3.json +++ b/builder/builder/builder_block_template/form_3/form_3.json @@ -1,14 +1,17 @@ { - "block": "{\"blockId\":\"6um6dfg30\",\"children\":[{\"blockId\":\"kv3hkfwvl\",\"children\":[{\"blockId\":\"a6gbqj3zl\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":1,\"overflow\":\"hidden\",\"position\":\"static\",\"width\":\"100%\",\"height\":\"32px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"First Name\"},\"classes\":[],\"dataKey\":null,\"element\":\"input\",\"customAttributes\":{\"name\":\"first_name\",\"required\":\"true\"}},{\"blockId\":\"za6939gew\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":1,\"overflow\":\"hidden\",\"position\":\"static\",\"width\":\"100%\",\"height\":\"32px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"Last Name\"},\"classes\":[],\"dataKey\":null,\"element\":\"input\",\"customAttributes\":{\"name\":\"last_name\",\"required\":\"true\"}}],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"row\",\"flexShrink\":1,\"height\":\"fit-content\",\"width\":\"100%\",\"overflow\":\"hidden\",\"position\":\"relative\",\"gap\":\"10px\",\"flexGrow\":0},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[],\"dataKey\":null,\"blockName\":\"container\",\"element\":\"div\",\"customAttributes\":{}},{\"blockId\":\"feg29l91n\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"width\":\"100%\",\"height\":\"32px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"Email\"},\"classes\":[],\"dataKey\":null,\"element\":\"input\",\"customAttributes\":{\"name\":\"email\",\"required\":\"true\"}},{\"blockId\":\"j267ivzos\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"top\":\"auto\",\"left\":\"auto\",\"width\":\"100%\",\"height\":\"150px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"Message\"},\"classes\":[],\"dataKey\":null,\"element\":\"textarea\",\"customAttributes\":{\"name\":\"message\",\"required\":\"true\"}},{\"blockId\":\"m68wwxf5e\",\"children\":[{\"blockId\":\"0aw9cg9y7\",\"children\":[],\"baseStyles\":{\"color\":\"var(--neutral-white, #FFF)\",\"fontSize\":\"14px\",\"fontWeight\":\"420\",\"height\":\"fit-content\",\"left\":\"auto\",\"letterSpacing\":\"0.28px\",\"lineHeight\":\"115%\",\"minWidth\":\"30px\",\"position\":\"static\",\"top\":\"auto\",\"width\":\"fit-content\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[\"__text_block__\"],\"dataKey\":null,\"element\":\"p\",\"innerHTML\":\"Submit
\",\"customAttributes\":{}}],\"baseStyles\":{\"background\":\"#171717\",\"borderRadius\":\"4px\",\"display\":\"flex\",\"flexDirection\":\"column\",\"height\":\"fit-content\",\"padding\":\"6px 8px\",\"width\":\"100%\",\"justifyContent\":\"flex-start\",\"alignItems\":\"center\"},\"rawStyles\":{\"flex-shrink\":\"0\",\"hover:background\":\"#383838\",\"transition\":\"all 0.1s ease-out\"},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[\"\"],\"dataKey\":null,\"element\":\"button\",\"customAttributes\":{}}],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"top\":\"auto\",\"left\":\"auto\",\"width\":\"100%\",\"paddingLeft\":\"20px\",\"paddingRight\":\"20px\",\"paddingBottom\":\"20px\",\"paddingTop\":\"20px\",\"maxWidth\":\"400px\",\"gap\":\"15px\",\"justifyContent\":\"flex-start\",\"alignItems\":\"center\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[],\"dataKey\":null,\"element\":\"form\",\"customAttributes\":{}}", - "category": "Basic", + "block": "{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"gap\": \"15px\",\"justifyContent\": \"flex-start\",\"left\": \"auto\",\"maxWidth\": \"400px\",\"overflow\": \"hidden\",\"paddingBottom\": \"20px\",\"paddingLeft\": \"20px\",\"paddingRight\": \"20px\",\"paddingTop\": \"20px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"100%\"},\"blockId\": \"6um6dfg30\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"row\",\"flexGrow\": 0,\"flexShrink\": 1,\"gap\": \"10px\",\"height\": \"fit-content\",\"overflow\": \"hidden\",\"position\": \"relative\",\"width\": \"100%\"},\"blockId\": \"kv3hkfwvl\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"placeholder\": \"First Name\"},\"baseStyles\": {\"borderColor\": \"#c9c9c9\",\"borderRadius\": \"4px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 1,\"fontSize\": \"14px\",\"height\": \"32px\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"a6gbqj3zl\",\"children\": [],\"classes\": [],\"customAttributes\": {\"name\": \"first_name\",\"required\": \"true\"},\"dataKey\": null,\"element\": \"input\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {\"placeholder\": \"Last Name\"},\"baseStyles\": {\"borderColor\": \"#c9c9c9\",\"borderRadius\": \"4px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 1,\"fontSize\": \"14px\",\"height\": \"32px\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"za6939gew\",\"children\": [],\"classes\": [],\"customAttributes\": {\"name\": \"last_name\",\"required\": \"true\"},\"dataKey\": null,\"element\": \"input\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {\"placeholder\": \"Email\"},\"baseStyles\": {\"borderColor\": \"#c9c9c9\",\"borderRadius\": \"4px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"fontSize\": \"14px\",\"height\": \"32px\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"feg29l91n\",\"children\": [],\"classes\": [],\"customAttributes\": {\"name\": \"email\",\"required\": \"true\"},\"dataKey\": null,\"element\": \"input\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {\"placeholder\": \"Message\"},\"baseStyles\": {\"borderColor\": \"#c9c9c9\",\"borderRadius\": \"4px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"fontSize\": \"14px\",\"height\": \"150px\",\"left\": \"auto\",\"overflow\": \"hidden\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"100%\"},\"blockId\": \"j267ivzos\",\"children\": [],\"classes\": [],\"customAttributes\": {\"name\": \"message\",\"required\": \"true\"},\"dataKey\": null,\"element\": \"textarea\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#171717\",\"borderRadius\": \"4px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"height\": \"fit-content\",\"justifyContent\": \"flex-start\",\"padding\": \"6px 8px\",\"width\": \"100%\"},\"blockId\": \"m68wwxf5e\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"var(--neutral-white, #FFF)\",\"fontSize\": \"14px\",\"fontWeight\": \"420\",\"height\": \"fit-content\",\"left\": \"auto\",\"letterSpacing\": \"0.28px\",\"lineHeight\": \"115%\",\"minWidth\": \"30px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"fit-content\"},\"blockId\": \"0aw9cg9y7\",\"children\": [],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Submit
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [\"\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"button\",\"mobileStyles\": {},\"rawStyles\": {\"flex-shrink\": \"0\",\"hover:background\": \"#383838\",\"transition\": \"all 0.1s ease-out\"},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"form\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Basic Forms", "creation": "2024-07-12 00:19:26.657647", "docstatus": 0, "doctype": "Block Template", "idx": 0, - "modified": "2024-08-05 10:08:41.880329", + "modified": "2024-09-19 13:12:17.932679", "modified_by": "Administrator", "name": "Form 3", + "order": 16, "owner": "Administrator", "preview": "/builder_assets/Form 3/form-3.png", + "preview_height": 1, + "preview_width": 1, "template_name": "Form 3" } \ No newline at end of file diff --git a/builder/builder/builder_block_template/heading/heading.json b/builder/builder/builder_block_template/heading/heading.json new file mode 100644 index 00000000..7bc24ce0 --- /dev/null +++ b/builder/builder/builder_block_template/heading/heading.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"fontSize\": \"2rem\",\"fontWeight\": \"bold\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"position\": \"static\",\"width\": \"fit-content\"},\"blockId\": \"sszefcous\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"h1\",\"innerHTML\": \"Heading\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Typography", + "creation": "2024-09-03 23:01:48.712698", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:09:29.235170", + "modified_by": "Administrator", + "name": "Heading", + "order": 10, + "owner": "Administrator", + "preview": "/builder_assets/Heading/heading.png", + "preview_height": 1, + "preview_width": 1, + "template_name": "Heading" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/hero_1/hero_1.json b/builder/builder/builder_block_template/hero_1/hero_1.json new file mode 100644 index 00000000..3ab04ec9 --- /dev/null +++ b/builder/builder/builder_block_template/hero_1/hero_1.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"gap\": \"10px\",\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"paddingBottom\": \"0px\",\"paddingTop\": \"0px\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"q7z15h823\",\"blockName\": \"hero\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"gap\": \"10px\",\"justifyContent\": \"center\",\"maxWidth\": \"1200px\",\"overflow\": \"hidden\",\"paddingBottom\": \"200px\",\"paddingTop\": \"200px\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"1w6wekyhh\",\"blockName\": \"wrapper\",\"children\": [{\"attributes\": {\"href\": \"/whats-new\"},\"baseStyles\": {\"alignItems\": \"center\",\"borderColor\": \"#EDEDED\",\"borderRadius\": \"100px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"paddingBottom\": \"4.5px\",\"paddingLeft\": \"8px\",\"paddingRight\": \"8px\",\"paddingTop\": \"4.5px\",\"position\": \"static\"},\"blockId\": \"pml2z8px1\",\"blockName\": \"whats-new\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"var(--text-icons-gray-6, #525252)\",\"fontFamily\": \"\",\"fontSize\": \"13px\",\"fontWeight\": \"400\",\"height\": \"fit-content\",\"letterSpacing\": \"0.065px\",\"lineHeight\": \"115%\",\"minWidth\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"l3okvvbip\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"What\\u2019s new
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"color\": \"#171717\",\"fontFamily\": \"\",\"fontSize\": \"48px\",\"fontWeight\": \"bold\",\"height\": \"fit-content\",\"letterSpacing\": \"0.48px\",\"lineHeight\": \"140%\",\"minWidth\": \"10px\",\"textAlign\": \"center\",\"width\": \"fit-content\"},\"blockId\": \"rdxw5m4ba\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"h1\",\"innerHTML\": \"Craft websites with striking style.\",\"mobileStyles\": {\"fontSize\": \"32px\",\"paddingLeft\": \"26px\",\"paddingRight\": \"26px\"},\"rawStyles\": {},\"tabletStyles\": {\"fontSize\": \"40px\"}},{\"attributes\": {},\"baseStyles\": {\"color\": \"#525252\",\"fontFamily\": \"\",\"fontSize\": \"16px\",\"fontWeight\": \"500\",\"height\": \"fit-content\",\"letterSpacing\": \"-0.08px\",\"lineHeight\": \"150%\",\"maxWidth\": \"518px\",\"minWidth\": \"10px\",\"textAlign\": \"center\",\"width\": \"fit-content\"},\"blockId\": \"z09gantla\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Transform your designs into stunning websites with Frappe builder, the ultimate web builder for creative pros.
\",\"mobileStyles\": {\"paddingLeft\": \"30px\",\"paddingRight\": \"30px\"},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"12px\",\"height\": \"fit-content\",\"marginTop\": \"26px\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"cd342xgme\",\"blockName\": \"actions\",\"children\": [{\"attributes\": {\"href\": \"/get-started\"},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#171717\",\"borderRadius\": \"12px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"height\": \"fit-content\",\"justifyContent\": \"center\",\"paddingBottom\": \"12px\",\"paddingLeft\": \"14px\",\"paddingRight\": \"14px\",\"paddingTop\": \"12px\",\"width\": \"fit-content\"},\"blockId\": \"a6fy7yivx\",\"blockName\": \"get-started\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"var(--neutral-white, #FFF)\",\"fontSize\": \"14px\",\"fontWeight\": \"420\",\"height\": \"fit-content\",\"left\": \"auto\",\"letterSpacing\": \"0.28px\",\"lineHeight\": \"115%\",\"minWidth\": \"30px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"fit-content\"},\"blockId\": \"9vliuvghj\",\"children\": [],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Get Started
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {\"flex-shrink\": \"0\",\"hover:background\": \"#383838\",\"transition\": \"all 0.1s ease-out\"},\"tabletStyles\": {\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\"}},{\"attributes\": {\"href\": \"/learn-more\"},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#F3F3F3\",\"borderRadius\": \"12px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"height\": \"fit-content\",\"justifyContent\": \"center\",\"paddingBottom\": \"12px\",\"paddingLeft\": \"14px\",\"paddingRight\": \"14px\",\"paddingTop\": \"12px\",\"width\": \"fit-content\"},\"blockId\": \"xglze3bl4\",\"blockName\": \"learn-more\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"#383838\",\"fontSize\": \"14px\",\"fontWeight\": \"420\",\"height\": \"fit-content\",\"left\": \"auto\",\"letterSpacing\": \"0.28px\",\"lineHeight\": \"115%\",\"minWidth\": \"30px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"fit-content\"},\"blockId\": \"0abw8dupb\",\"children\": [],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Learn More
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {\"flex-shrink\": \"0\",\"hover:background\": \"#383838\",\"transition\": \"all 0.1s ease-out\"},\"tabletStyles\": {\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Structure", + "creation": "2024-09-17 12:24:13.824273", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:08:25.812814", + "modified_by": "Administrator", + "name": "Hero 1", + "order": 4, + "owner": "Administrator", + "preview": "/builder_assets/Hero 1/hero_1.png", + "preview_height": 2, + "preview_width": 2, + "template_name": "Hero 1" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/hero_2/hero_2.json b/builder/builder/builder_block_template/hero_2/hero_2.json new file mode 100644 index 00000000..bd6a1803 --- /dev/null +++ b/builder/builder/builder_block_template/hero_2/hero_2.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"position\": \"relative\",\"width\": \"100%\"},\"blockId\": \"xiuhb9ky8\",\"blockName\": \"hero\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"16px\",\"justifyContent\": \"space-between\",\"maxWidth\": \"1200px\",\"overflow\": \"hidden\",\"paddingBottom\": \"120px\",\"paddingLeft\": \"80px\",\"paddingRight\": \"0px\",\"paddingTop\": \"120px\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"q7z15h823\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"gap\": \"10px\",\"height\": \"fit-content\",\"overflow\": \"hidden\",\"position\": \"relative\",\"width\": \"fit-content\"},\"blockId\": \"vhdziphwj\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"href\": \"/whats-new\"},\"baseStyles\": {\"alignItems\": \"center\",\"borderColor\": \"#EDEDED\",\"borderRadius\": \"100px\",\"borderStyle\": \"solid\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"paddingBottom\": \"4.5px\",\"paddingLeft\": \"8px\",\"paddingRight\": \"8px\",\"paddingTop\": \"4.5px\",\"position\": \"static\",\"width\": \"fit-content\"},\"blockId\": \"m9j6t0ttk\",\"blockName\": \"whats-new\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"var(--text-icons-gray-6, #525252)\",\"fontFamily\": \"\",\"fontSize\": \"13px\",\"fontWeight\": \"400\",\"height\": \"fit-content\",\"letterSpacing\": \"0.065px\",\"lineHeight\": \"115%\",\"minWidth\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"8ahpfu1ke\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"What\\u2019s new
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"color\": \"#171717\",\"fontFamily\": \"\",\"fontSize\": \"48px\",\"fontWeight\": \"700\",\"height\": \"fit-content\",\"letterSpacing\": \"0.48px\",\"lineHeight\": \"104%\",\"maxWidth\": \"450px\",\"minWidth\": \"10px\",\"textAlign\": \"left\",\"width\": \"fit-content\"},\"blockId\": \"hhltxd6fl\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"h1\",\"innerHTML\": \"Craft websites with striking style.\",\"mobileStyles\": {\"fontSize\": \"34px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\"},\"rawStyles\": {},\"tabletStyles\": {\"fontSize\": \"40px\",\"maxWidth\": \"300px\"}},{\"attributes\": {},\"baseStyles\": {\"color\": \"#525252\",\"fontFamily\": \"\",\"fontSize\": \"16px\",\"fontWeight\": \"500\",\"height\": \"fit-content\",\"letterSpacing\": \"-0.08px\",\"lineHeight\": \"150%\",\"maxWidth\": \"350px\",\"minWidth\": \"10px\",\"textAlign\": \"left\",\"width\": \"fit-content\"},\"blockId\": \"bjdfr96pk\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Transform your designs into stunning websites with Frappe builder, the ultimate web builder for creative pros.
\",\"mobileStyles\": {\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\"},\"rawStyles\": {},\"tabletStyles\": {\"maxWidth\": \"300px\"}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"12px\",\"height\": \"fit-content\",\"marginTop\": \"15px\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"0swftvl8l\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"href\": \"/get-started\"},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#171717\",\"borderRadius\": \"12px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"height\": \"fit-content\",\"justifyContent\": \"center\",\"paddingBottom\": \"12px\",\"paddingLeft\": \"14px\",\"paddingRight\": \"14px\",\"paddingTop\": \"12px\",\"width\": \"fit-content\"},\"blockId\": \"vij8cbaa3\",\"blockName\": \"get-started\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"var(--neutral-white, #FFF)\",\"fontSize\": \"14px\",\"fontWeight\": \"420\",\"height\": \"fit-content\",\"left\": \"auto\",\"letterSpacing\": \"0.28px\",\"lineHeight\": \"115%\",\"minWidth\": \"30px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"fit-content\"},\"blockId\": \"si77pxsal\",\"children\": [],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Get Started
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {\"flex-shrink\": \"0\",\"hover:background\": \"#383838\",\"transition\": \"all 0.1s ease-out\"},\"tabletStyles\": {\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\"}},{\"attributes\": {\"href\": \"/learn-more\"},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#F3F3F3\",\"borderRadius\": \"12px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"height\": \"fit-content\",\"justifyContent\": \"center\",\"paddingBottom\": \"12px\",\"paddingLeft\": \"14px\",\"paddingRight\": \"14px\",\"paddingTop\": \"12px\",\"width\": \"fit-content\"},\"blockId\": \"ly49oj9jh\",\"blockName\": \"learn-more\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"#383838\",\"fontSize\": \"14px\",\"fontWeight\": \"420\",\"height\": \"fit-content\",\"left\": \"auto\",\"letterSpacing\": \"0.28px\",\"lineHeight\": \"115%\",\"minWidth\": \"30px\",\"position\": \"static\",\"top\": \"auto\",\"width\": \"fit-content\"},\"blockId\": \"g2x4nidta\",\"children\": [],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Learn More
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [\"__text_block__\"],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {\"flex-shrink\": \"0\",\"hover:background\": \"#383838\",\"transition\": \"all 0.1s ease-out\"},\"tabletStyles\": {\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {\"src\": \"/builder_assets/Hero 2/image0ccb3c.webp\"},\"baseStyles\": {\"borderRadius\": \"50px\",\"flexShrink\": 0,\"objectFit\": \"cover\"},\"blockId\": \"5irh6smpx\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"img\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {\"alignItems\": \"flex-start\",\"flexDirection\": \"column\",\"gap\": \"40px\",\"justifyContent\": \"center\",\"paddingBottom\": \"120px\",\"paddingLeft\": \"20px\",\"paddingRight\": \"20px\"},\"rawStyles\": {},\"tabletStyles\": {\"paddingLeft\": \"60px\",\"paddingRight\": \"60px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Structure", + "creation": "2024-09-17 14:08:36.529314", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:08:38.218157", + "modified_by": "Administrator", + "name": "Hero 2", + "order": 5, + "owner": "Administrator", + "preview": "/builder_assets/Hero 2/hero_2.png", + "preview_height": 2, + "preview_width": 2, + "template_name": "Hero 2" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/label/label.json b/builder/builder/builder_block_template/label/label.json new file mode 100644 index 00000000..0e3dbc1d --- /dev/null +++ b/builder/builder/builder_block_template/label/label.json @@ -0,0 +1,14 @@ +{ + "block": "{\"blockId\":\"pjw5akr39\",\"children\":[{\"blockId\":\"xi48r2pme\",\"children\":[],\"baseStyles\":{\"fontSize\":\"14px\",\"width\":\"fit-content\",\"height\":\"fit-content\",\"lineHeight\":\"115%\",\"minWidth\":\"10px\",\"position\":\"static\",\"top\":\"auto\",\"left\":\"auto\",\"fontFamily\":\"\",\"fontWeight\":\"400\",\"letterSpacing\":\"0.07px\",\"color\":\"#7C7C7C\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[],\"dataKey\":null,\"element\":\"label\",\"innerHTML\":\"Label
\",\"customAttributes\":{\"for\":\"name\"}},{\"blockId\":\"ki103x8w7\",\"children\":[],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"overflow\":\"hidden\",\"position\":\"static\",\"width\":\"100%\",\"height\":\"32px\",\"borderRadius\":\"4px\",\"borderColor\":\"#c9c9c9\",\"borderWidth\":\"1px\",\"borderStyle\":\"solid\",\"fontSize\":\"14px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"placeholder\":\"Name\"},\"classes\":[],\"dataKey\":null,\"element\":\"input\",\"customAttributes\":{\"name\":\"name\",\"required\":\"true\"}}],\"baseStyles\":{\"display\":\"flex\",\"flexDirection\":\"column\",\"flexShrink\":0,\"height\":\"fit-content\",\"width\":\"100%\",\"overflow\":\"hidden\",\"position\":\"relative\",\"gap\":\"5px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{},\"classes\":[],\"dataKey\":null,\"blockName\":\"container\",\"element\":\"div\",\"customAttributes\":{}}", + "category": "Form parts", + "creation": "2024-09-03 23:00:16.365741", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-03 23:00:16.365741", + "modified_by": "Administrator", + "name": "Label", + "owner": "Administrator", + "preview": "/builder_assets/Label/label.png", + "template_name": "Label" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/navbar_1/navbar_1.json b/builder/builder/builder_block_template/navbar_1/navbar_1.json new file mode 100644 index 00000000..0dfcd882 --- /dev/null +++ b/builder/builder/builder_block_template/navbar_1/navbar_1.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"boxShadow\": \"rgba(0, 0, 0, 0) 0px 0px 0px 0px, rgba(0, 0, 0, 0) 0px 0px 0px 0px, rgba(0, 0, 0, 0.05) 0px 1px 2px 0px, rgba(0, 0, 0, 0.05) 0px 1px 3px 0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"y2d301ae6\",\"blockName\": \"navbar\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"boxShadow\": \"None\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"height\": \"fit-content\",\"justifyContent\": \"space-between\",\"maxWidth\": \"1200px\",\"overflow\": \"hidden\",\"paddingBottom\": \"16px\",\"paddingLeft\": \"36px\",\"paddingRight\": \"36px\",\"paddingTop\": \"16px\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"0vtlpu03s\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"href\": \"/home\"},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"0jmcvjcsj\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"src\": \"/builder_assets/Navbar 1/imageca9535.webp\"},\"baseStyles\": {\"flexShrink\": 0,\"objectFit\": \"cover\",\"width\": \"90px\"},\"blockId\": \"bsrrts4ka\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"img\",\"mobileStyles\": {\"display\": \"none\"},\"rawStyles\": {},\"tabletStyles\": {\"width\": \"80px\"}},{\"attributes\": {\"src\": \"/builder_assets/Navbar 1/Logo.webp\"},\"baseStyles\": {\"display\": \"none\",\"flexShrink\": 0,\"objectFit\": \"cover\"},\"blockId\": \"hoqwsctwv\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"img\",\"mobileStyles\": {\"display\": \"flex\",\"width\": \"30px\"},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"4px\",\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"9m6ziw1vz\",\"children\": [{\"attributes\": {\"href\": \"/home\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"ek6bh4o1y\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Home
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/product\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"30sxa7qpb\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Product
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/features\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"oxe8p96l8\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Features
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/about\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"490v0w3do\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"About us
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/pricing\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"tfp4aede9\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Pricing
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"nav\",\"mobileStyles\": {\"__last_display\": \"flex\",\"display\": \"none\"},\"rawStyles\": {},\"tabletStyles\": {\"gap\": \"0px\"}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"8px\",\"height\": \"fit-content\",\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"9s9e7v41r\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"href\": \"/login\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"zf0y11fvx\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Log in
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/get-started\"},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"color(display-p3 0.090 0.090 0.090)\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\"},\"blockId\": \"tlh5pzpsg\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"color(display-p3 1.000 1.000 1.000)\",\"fontFamily\": \"\",\"fontSize\": \"16px\",\"fontWeight\": \"500\",\"height\": \"fit-content\",\"letterSpacing\": \"0.005em\",\"lineHeight\": \"115%\",\"minWidth\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"tmf6exowl\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Get started
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {\"__last_display\": \"flex\",\"display\": \"none\"},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"__last_display\": \"flex\",\"display\": \"none\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"c70gdumv2\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"height\": \"18px\",\"width\": \"18px\"},\"blockId\": \"l1fodmqkq\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"button\",\"mobileStyles\": {\"display\": \"flex\",\"paddingBottom\": \"7px\",\"paddingLeft\": \"7px\",\"paddingRight\": \"7px\",\"paddingTop\": \"7px\"},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {\"paddingLeft\": \"16px\",\"paddingRight\": \"16px\"},\"rawStyles\": {},\"tabletStyles\": {\"paddingLeft\": \"16px\",\"paddingRight\": \"16px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"editable\": false,\"element\": \"header\",\"mobileStyles\": {\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\"},\"rawStyles\": {},\"tabletStyles\": {\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\"}}", + "category": "Structure", + "creation": "2024-09-17 11:48:14.626645", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:08:11.964111", + "modified_by": "Administrator", + "name": "Navbar 1", + "order": 2, + "owner": "Administrator", + "preview": "/builder_assets/Navbar 1/navbar_1.png", + "preview_height": 1, + "preview_width": 2, + "template_name": "Navbar 1" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/navbar_2/navbar_2.json b/builder/builder/builder_block_template/navbar_2/navbar_2.json new file mode 100644 index 00000000..b9337b86 --- /dev/null +++ b/builder/builder/builder_block_template/navbar_2/navbar_2.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"boxShadow\": \"rgba(0, 0, 0, 0) 0px 0px 0px 0px, rgba(0, 0, 0, 0) 0px 0px 0px 0px, rgba(0, 0, 0, 0.05) 0px 1px 2px 0px, rgba(0, 0, 0, 0.05) 0px 1px 3px 0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"go755hiqz\",\"blockName\": \"hero\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"boxShadow\": \"None\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"justifyContent\": \"space-between\",\"maxWidth\": \"1200px\",\"overflow\": \"hidden\",\"paddingBottom\": \"16px\",\"paddingLeft\": \"36px\",\"paddingRight\": \"36px\",\"paddingTop\": \"16px\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"kzbg33fba\",\"children\": [{\"attributes\": {\"href\": \"/home\"},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"40px\",\"height\": \"fit-content\",\"justifyContent\": \"flex-start\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"zknkfszm7\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"src\": \"/builder_assets/Navbar 2/Logo.webp\"},\"baseStyles\": {\"flexShrink\": 0,\"height\": \"30px\",\"objectFit\": \"cover\",\"width\": \"30px\"},\"blockId\": \"uew56afyp\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"img\",\"mobileStyles\": {\"display\": \"flex\"},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"4px\",\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"qrq7fgnw3\",\"children\": [{\"attributes\": {\"href\": \"/home\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"onijv3a3m\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Home
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/product\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"28gj17j17\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Product
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/features\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"geznu05ij\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Features
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/about\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"mp4q703ic\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"About us
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/pricing\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"dbr8zdxrg\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Pricing
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"nav\",\"mobileStyles\": {\"__last_display\": \"flex\",\"display\": \"none\"},\"rawStyles\": {},\"tabletStyles\": {\"gap\": \"0px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"gap\": \"30px\"}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"8px\",\"height\": \"fit-content\",\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"s2m739lp7\",\"blockName\": \"container\",\"children\": [{\"attributes\": {\"href\": \"/login\"},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"l9v23eaw4\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"innerHTML\": \"Log in
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {\"padding\": \"10px\"}},{\"attributes\": {\"href\": \"/get-started\"},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"color(display-p3 0.090 0.090 0.090)\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"12px\",\"paddingRight\": \"12px\",\"paddingTop\": \"10px\"},\"blockId\": \"ni08tqcr9\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"color\": \"color(display-p3 1.000 1.000 1.000)\",\"fontFamily\": \"\",\"fontSize\": \"16px\",\"fontWeight\": \"500\",\"height\": \"fit-content\",\"letterSpacing\": \"0.005em\",\"lineHeight\": \"115%\",\"minWidth\": \"10px\",\"width\": \"fit-content\"},\"blockId\": \"98oddel44\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Get started
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"a\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {\"__last_display\": \"flex\",\"display\": \"none\"},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"__last_display\": \"flex\",\"display\": \"none\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"overflow\": \"hidden\",\"width\": \"fit-content\"},\"blockId\": \"vydncgo65\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"height\": \"18px\",\"width\": \"18px\"},\"blockId\": \"ae7k6drur\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"button\",\"mobileStyles\": {\"display\": \"flex\",\"paddingBottom\": \"7px\",\"paddingLeft\": \"7px\",\"paddingRight\": \"7px\",\"paddingTop\": \"7px\"},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": {\"key\": \"\",\"property\": \"\",\"type\": \"\"},\"element\": \"div\",\"mobileStyles\": {\"paddingLeft\": \"16px\",\"paddingRight\": \"16px\"},\"rawStyles\": {},\"tabletStyles\": {\"paddingLeft\": \"16px\",\"paddingRight\": \"16px\"}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": {\"key\": \"\",\"property\": \"\",\"type\": \"\"},\"element\": \"header\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Structure", + "creation": "2024-09-17 11:44:33.077409", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-29 21:08:20.795780", + "modified_by": "Administrator", + "name": "Navbar 2", + "order": 3, + "owner": "Administrator", + "preview": "/builder_assets/Navbar 2/navbar_2.png", + "preview_height": 1, + "preview_width": 2, + "template_name": "Navbar 2" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/paragraph/paragraph.json b/builder/builder/builder_block_template/paragraph/paragraph.json new file mode 100644 index 00000000..644d3119 --- /dev/null +++ b/builder/builder/builder_block_template/paragraph/paragraph.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"position\": \"static\",\"width\": \"fit-content\"},\"blockId\": \"z5hw6riip\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"\\\"Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.\\\"
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Typography", + "creation": "2024-09-03 23:03:27.782586", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:09:32.402504", + "modified_by": "Administrator", + "name": "Paragraph", + "order": 11, + "owner": "Administrator", + "preview": "/builder_assets/Paragraph/paragraph.png", + "preview_height": 1, + "preview_width": 1, + "template_name": "Paragraph" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/quick_grid/quick_grid.json b/builder/builder/builder_block_template/quick_grid/quick_grid.json new file mode 100644 index 00000000..9bbabd85 --- /dev/null +++ b/builder/builder/builder_block_template/quick_grid/quick_grid.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"display\": \"grid\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"gap\": \"20px\",\"gridTemplateColumns\": \"repeat(2, minmax(200px, 1fr))\",\"height\": \"800px\",\"overflow\": \"hidden\",\"padding\": \"20px\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"9jln72b1t\",\"blockName\": \"grid\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"background\": \"#e2e2e2\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"100%\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"auto\"},\"blockId\": \"vwf92j0d4\",\"blockName\": \"cell-1\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"background\": \"#e2e2e2\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"100%\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"auto\"},\"blockId\": \"2nsk5x26t\",\"blockName\": \"cell-2\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"background\": \"#e2e2e2\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"100%\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"auto\"},\"blockId\": \"z19h14huq\",\"blockName\": \"cell-3\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"background\": \"#e2e2e2\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"100%\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"auto\"},\"blockId\": \"7ya6tzprf\",\"blockName\": \"cell-4\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Structure", + "creation": "2024-09-19 13:01:06.663112", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:09:25.446243", + "modified_by": "Administrator", + "name": "Quick Grid", + "order": 9, + "owner": "Administrator", + "preview": "/builder_assets/Quick Grid/quick-grid.png", + "preview_height": 1, + "preview_width": 1, + "template_name": "Quick Grid" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/quick_stack/quick_stack.json b/builder/builder/builder_block_template/quick_stack/quick_stack.json new file mode 100644 index 00000000..3f3216bc --- /dev/null +++ b/builder/builder/builder_block_template/quick_stack/quick_stack.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"background\": \"#ffffff\",\"display\": \"flex\",\"flexDirection\": \"row\",\"flexShrink\": 0,\"gap\": \"20px\",\"height\": \"600px\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"1w8x9wtvc\",\"blockName\": \"Stack\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#e2e2e2\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 1,\"height\": \"100%\",\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"xh8ticga0\",\"blockName\": \"container\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 1,\"gap\": \"20px\",\"height\": \"100%\",\"overflow\": \"hidden\",\"position\": \"relative\",\"width\": \"100%\"},\"blockId\": \"dqkd8jjr8\",\"blockName\": \"container\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#e2e2e2\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 1,\"height\": \"100%\",\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"8xas835ea\",\"blockName\": \"container\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"#e2e2e2\",\"borderRadius\": \"10px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 1,\"height\": \"100%\",\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"position\": \"static\",\"width\": \"100%\"},\"blockId\": \"sntr9rcir\",\"blockName\": \"container\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"editable\": false,\"element\": \"div\",\"mobileStyles\": {\"flexDirection\": \"column\"},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Structure", + "creation": "2024-09-03 22:36:28.203260", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:35:31.530861", + "modified_by": "Administrator", + "name": "Quick Stack", + "order": 8, + "owner": "Administrator", + "preview": "/builder_assets/Quick Stack/quick-stack.png", + "preview_height": 1, + "preview_width": 1, + "template_name": "Quick Stack" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/testimonial_1/testimonial_1.json b/builder/builder/builder_block_template/testimonial_1/testimonial_1.json new file mode 100644 index 00000000..07ae9762 --- /dev/null +++ b/builder/builder/builder_block_template/testimonial_1/testimonial_1.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"display\": \"flex\",\"flexDirection\": \"column\",\"flexShrink\": 0,\"height\": \"fit-content\",\"justifyContent\": \"center\",\"overflow\": \"hidden\",\"width\": \"100%\"},\"blockId\": \"80gz6xaq2\",\"blockName\": \"testimonial-wrapper\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"rgb(255, 255, 255)\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"justifyContent\": \"space-between\",\"maxWidth\": \"1200px\",\"paddingBottom\": \"70px\",\"paddingLeft\": \"40px\",\"paddingRight\": \"40px\",\"paddingTop\": \"70px\",\"width\": \"100%\"},\"blockId\": \"uoi8pxczq\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"rgb(255, 255, 255)\",\"borderColor\": \"rgb(237, 237, 237)\",\"borderRadius\": \"34px\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"6px\",\"justifyContent\": \"center\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"10px\",\"paddingRight\": \"10px\",\"paddingTop\": \"10px\"},\"blockId\": \"wfk91yvhp\",\"blockName\": \"prev-button\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"height\": \"21px\",\"width\": \"20px\"},\"blockId\": \"iew39254n\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"button\",\"innerHTML\": \"\",\"mobileStyles\": {\"paddingBottom\": \"5px\",\"paddingLeft\": \"5px\",\"paddingRight\": \"5px\",\"paddingTop\": \"5px\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"72px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"eakp683av\",\"blockName\": \"content\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"40px\",\"justifyContent\": \"flex-start\",\"maxWidth\": \"560px\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"g44wdzdfd\",\"blockName\": \"testimony\",\"children\": [{\"attributes\": {\"src\": \"/builder_assets/Testimonial 1/imagebab78c.webp\"},\"baseStyles\": {\"background\": \"url(image.png)\",\"flexShrink\": 0,\"height\": \"35px\",\"objectFit\": \"cover\",\"width\": \"104px\"},\"blockId\": \"vnpylp2pu\",\"blockName\": \"logo\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"img\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(23, 23, 23)\",\"display\": \"flex\",\"fontSize\": \"24px\",\"letterSpacing\": \"0px\",\"lineHeight\": \"139.9999976158142%\",\"textAlign\": \"center\"},\"blockId\": \"7ps8gs1gs\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"A world-class design system project is currently underway. I've been fortunate enough to beta test it. Keep tracking this - it might turn into the perfect design system for your upcoming side project or new SaaS platform.
\",\"mobileStyles\": {\"color\": \"color(display-p3 0.090 0.090 0.090)\",\"fontSize\": \"18px\",\"fontWeight\": \"500\",\"letterSpacing\": \"-0.01em\",\"lineHeight\": \"150%\",\"textAlign\": \"center\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"borderRadius\": \"0px\",\"display\": \"flex\",\"flexDirection\": \"column\",\"gap\": \"4px\",\"justifyContent\": \"flex-start\",\"paddingBottom\": \"0px\",\"paddingLeft\": \"0px\",\"paddingRight\": \"0px\",\"paddingTop\": \"0px\"},\"blockId\": \"i88odt6fm\",\"blockName\": \"testimony-by\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(23, 23, 23)\",\"display\": \"flex\",\"fontSize\": \"18px\",\"letterSpacing\": \"1%\",\"lineHeight\": \"114.99999761581421%\",\"textAlign\": \"center\"},\"blockId\": \"b5ohij9kn\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Lynn Tanner
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"color\": \"rgb(82, 82, 82)\",\"display\": \"flex\",\"fontSize\": \"14px\",\"letterSpacing\": \"0%\",\"lineHeight\": \"150%\",\"textAlign\": \"center\"},\"blockId\": \"fdjz2ork4\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Chief Technology Officer, Notion
\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}},{\"attributes\": {},\"baseStyles\": {\"alignItems\": \"center\",\"background\": \"rgb(255, 255, 255)\",\"borderColor\": \"rgb(237, 237, 237)\",\"borderRadius\": \"100px\",\"borderWidth\": \"1px\",\"display\": \"flex\",\"flexDirection\": \"row\",\"gap\": \"6px\",\"justifyContent\": \"center\",\"paddingBottom\": \"10px\",\"paddingLeft\": \"10px\",\"paddingRight\": \"10px\",\"paddingTop\": \"10px\"},\"blockId\": \"nq0wglh9f\",\"blockName\": \"next-button\",\"children\": [{\"attributes\": {},\"baseStyles\": {\"height\": \"21px\",\"width\": \"20px\"},\"blockId\": \"zblz7t7ov\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"innerHTML\": \"\",\"mobileStyles\": {},\"originalElement\": \"__raw_html__\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"button\",\"innerHTML\": \"\",\"mobileStyles\": {\"paddingBottom\": \"5px\",\"paddingLeft\": \"5px\",\"paddingRight\": \"5px\",\"paddingTop\": \"5px\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"section\",\"innerHTML\": \"\",\"mobileStyles\": {\"paddingLeft\": \"30px\",\"paddingRight\": \"30px\"},\"originalElement\": \"\",\"rawStyles\": {},\"tabletStyles\": {}}],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"div\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Structure", + "creation": "2024-09-18 19:38:39.294613", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-10-14 00:05:16.066806", + "modified_by": "Administrator", + "name": "Testimonial 1", + "order": 6, + "owner": "Administrator", + "preview": "/builder_assets/Testimonial 1/testimonial_1.png", + "preview_height": 2, + "preview_width": 2, + "template_name": "Testimonial 1" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/text_link/text_link.json b/builder/builder/builder_block_template/text_link/text_link.json new file mode 100644 index 00000000..a6792431 --- /dev/null +++ b/builder/builder/builder_block_template/text_link/text_link.json @@ -0,0 +1,17 @@ +{ + "block": "{\"attributes\": {},\"baseStyles\": {\"fontSize\": \"16px\",\"height\": \"fit-content\",\"lineHeight\": \"1.4\",\"minWidth\": \"10px\",\"position\": \"static\",\"width\": \"fit-content\"},\"blockId\": \"z5hw6riip\",\"children\": [],\"classes\": [],\"customAttributes\": {},\"dataKey\": null,\"element\": \"p\",\"innerHTML\": \"Hi, click me
\",\"mobileStyles\": {},\"rawStyles\": {},\"tabletStyles\": {}}", + "category": "Typography", + "creation": "2024-09-03 23:08:18.568344", + "docstatus": 0, + "doctype": "Block Template", + "idx": 0, + "modified": "2024-09-19 13:09:34.940528", + "modified_by": "Administrator", + "name": "Text Link", + "order": 12, + "owner": "Administrator", + "preview": "/builder_assets/Text Link/text-link.png", + "preview_height": 1, + "preview_width": 1, + "template_name": "Text Link" +} \ No newline at end of file diff --git a/builder/builder/builder_block_template/video/video.json b/builder/builder/builder_block_template/video/video.json index 98fbdebf..721f4934 100644 --- a/builder/builder/builder_block_template/video/video.json +++ b/builder/builder/builder_block_template/video/video.json @@ -1,11 +1,11 @@ { "block": "{\"blockId\":\"69xrb0gy4\",\"children\":[],\"baseStyles\":{\"objectFit\":\"cover\",\"width\":\"550px\"},\"rawStyles\":{},\"mobileStyles\":{},\"tabletStyles\":{},\"attributes\":{\"autoplay\":\"\",\"muted\":\"\",\"src\":\"https://cdn.free-stock.video/2152023/wave-nature-waves-ocean-water-sand-water-23350-small.mp4\"},\"classes\":[],\"dataKey\":null,\"element\":\"video\",\"customAttributes\":{}}", - "category": "Basic", + "category": "Media", "creation": "2024-07-10 23:09:39.980242", "docstatus": 0, "doctype": "Block Template", "idx": 0, - "modified": "2024-07-12 09:34:20.763472", + "modified": "2024-09-03 22:16:17.757615", "modified_by": "Administrator", "name": "Video", "owner": "Administrator", diff --git a/builder/builder/doctype/block_template/block_template.json b/builder/builder/doctype/block_template/block_template.json index 0c469df7..e1b8e006 100644 --- a/builder/builder/doctype/block_template/block_template.json +++ b/builder/builder/doctype/block_template/block_template.json @@ -9,7 +9,10 @@ "template_name", "block", "preview", - "category" + "preview_width", + "preview_height", + "category", + "order" ], "fields": [ { @@ -39,13 +42,31 @@ "fieldtype": "Select", "in_list_view": 1, "label": "Category", - "options": "Basic\nStructure" + "options": "Structure\nBasic\nTypography\nBasic Forms\nForm parts\nMedia\nAdvanced", + "search_index": 1 + }, + { + "default": "1", + "fieldname": "preview_width", + "fieldtype": "Int", + "label": "Preview Width" + }, + { + "default": "1", + "fieldname": "preview_height", + "fieldtype": "Int", + "label": "Preview Height" + }, + { + "fieldname": "order", + "fieldtype": "Int", + "label": "Order" } ], "in_create": 1, "index_web_pages_for_search": 1, "links": [], - "modified": "2024-08-22 21:48:45.695550", + "modified": "2024-09-19 13:07:00.935349", "modified_by": "Administrator", "module": "Builder", "name": "Block Template", diff --git a/builder/builder/doctype/block_template/block_template.py b/builder/builder/doctype/block_template/block_template.py index ac82d6d6..02d51d3b 100644 --- a/builder/builder/doctype/block_template/block_template.py +++ b/builder/builder/doctype/block_template/block_template.py @@ -11,6 +11,7 @@ from frappe.modules.export_file import export_to_files from builder.builder.doctype.builder_page.builder_page import get_template_assets_folder_path +from builder.utils import copy_img_to_asset_folder class BlockTemplate(Document): @@ -26,6 +27,11 @@ def on_update(self): self.preview = f"/builder_assets/{self.name}/{self.preview.split('/')[-1]}" self.db_set("preview", self.preview) + block = frappe.parse_json(self.block) + if block: + copy_img_to_asset_folder(block, self) + self.db_set("block", frappe.as_json(block, indent=None)) + export_to_files( record_list=[ [ diff --git a/builder/builder/doctype/builder_client_script/builder_client_script.py b/builder/builder/doctype/builder_client_script/builder_client_script.py index 30d84688..a5bd6516 100644 --- a/builder/builder/doctype/builder_client_script/builder_client_script.py +++ b/builder/builder/doctype/builder_client_script/builder_client_script.py @@ -4,9 +4,11 @@ import os import frappe +from csscompressor import compress from frappe.model.document import Document from frappe.modules.export_file import export_to_files from frappe.utils import get_files_path +from jsmin import jsmin class BuilderClientScript(Document): @@ -32,7 +34,12 @@ def update_script_file(self): file_path = get_files_path(f"{folder_name}/{file_name}") os.makedirs(os.path.dirname(file_path), exist_ok=True) with open(file_path, "w") as f: - f.write(self.script) + script = self.script or "" + if script_type == "JavaScript": + script = jsmin(script, quote_chars="'\"`") + if script_type == "CSS": + script = compress(script) + f.write(script) public_url = f"/files/{folder_name}/{file_name}?v={frappe.generate_hash(length=10)}" self.db_set("public_url", public_url, commit=True) diff --git a/builder/builder/doctype/builder_client_script/patches/trigger_asset_compression.py b/builder/builder/doctype/builder_client_script/patches/trigger_asset_compression.py new file mode 100644 index 00000000..fbac24c6 --- /dev/null +++ b/builder/builder/doctype/builder_client_script/patches/trigger_asset_compression.py @@ -0,0 +1,11 @@ +import frappe + + +def execute(): + for doc in frappe.get_all("Builder Client Script"): + script_doc = frappe.get_doc("Builder Client Script", doc.name) + try: + script_doc.on_update() # this will compress script and save it to file + except Exception as e: + print(f"Error compressing script for {script_doc.name}: {e}") + continue diff --git a/builder/builder/doctype/builder_page/builder_page.json b/builder/builder/doctype/builder_page/builder_page.json index c08c929b..3c8e69a4 100644 --- a/builder/builder/doctype/builder_page/builder_page.json +++ b/builder/builder/doctype/builder_page/builder_page.json @@ -32,7 +32,10 @@ "page_title", "meta_description", "meta_image", - "set_meta_tags" + "set_meta_tags", + "options_tab", + "authenticated_access", + "disable_indexing" ], "fields": [ { @@ -172,11 +175,30 @@ { "fieldname": "column_break_ymjg", "fieldtype": "Column Break" + }, + { + "fieldname": "options_tab", + "fieldtype": "Tab Break", + "label": "Options" + }, + { + "default": "0", + "description": "Only allow logged-in users to view this page.", + "fieldname": "authenticated_access", + "fieldtype": "Check", + "label": "Authenticated Access" + }, + { + "default": "0", + "description": "Prevent search engines from indexing this page", + "fieldname": "disable_indexing", + "fieldtype": "Check", + "label": "Disable Indexing" } ], "index_web_pages_for_search": 1, "links": [], - "modified": "2024-07-02 18:13:15.327787", + "modified": "2024-09-02 14:20:24.457766", "modified_by": "Administrator", "module": "Builder", "name": "Builder Page", diff --git a/builder/builder/doctype/builder_page/builder_page.py b/builder/builder/doctype/builder_page/builder_page.py index d4cdf7d3..1ee8a6d7 100644 --- a/builder/builder/doctype/builder_page/builder_page.py +++ b/builder/builder/doctype/builder_page/builder_page.py @@ -2,24 +2,16 @@ # For license information, please see license.txt import os -import re import shutil -from io import BytesIO -from urllib.parse import unquote import bs4 as bs import frappe import frappe.utils -import requests -from frappe.core.doctype.file.file import get_local_image -from frappe.core.doctype.file.utils import delete_file -from frappe.model.document import Document from frappe.modules import scrub from frappe.modules.export_file import export_to_files from frappe.utils.caching import redis_cache from frappe.utils.jinja import render_template from frappe.utils.safe_exec import is_safe_exec_enabled, safe_exec -from frappe.utils.telemetry import capture from frappe.website.page_renderers.document_page import DocumentPage from frappe.website.path_resolver import evaluate_dynamic_routes from frappe.website.path_resolver import resolve_path as original_resolve_path @@ -27,12 +19,20 @@ from frappe.website.utils import clear_cache from frappe.website.website_generator import WebsiteGenerator from jinja2.exceptions import TemplateSyntaxError -from PIL import Image from werkzeug.routing import Rule from builder.hooks import builder_path from builder.html_preview_image import generate_preview -from builder.utils import safer_exec +from builder.utils import ( + ColonRule, + camel_case_to_kebab_case, + copy_img_to_asset_folder, + escape_single_quotes, + execute_script, + get_builder_page_preview_paths, + get_template_assets_folder_path, + is_component_used, +) MOBILE_BREAKPOINT = 576 TABLET_BREAKPOINT = 768 @@ -44,16 +44,27 @@ def can_render(self): if page := find_page_with_path(self.path): self.doctype = "Builder Page" self.docname = page + self.validate_access() return True for d in get_web_pages_with_dynamic_routes(): - if evaluate_dynamic_routes([Rule(f"/{d.route}", endpoint=d.name)], self.path): - self.doctype = "Builder Page" - self.docname = d.name - return True + try: + if evaluate_dynamic_routes([ColonRule(f"/{d.route}", endpoint=d.name)], self.path): + self.doctype = "Builder Page" + self.docname = d.name + self.validate_access() + return True + except ValueError: + return False return False + def validate_access(self): + if self.docname: + self.doc = frappe.get_cached_doc(self.doctype, self.docname) + if self.doc.authenticated_access and frappe.session.user == "Guest": + raise frappe.PermissionError("Please log in to view this page.") + class BuilderPage(WebsiteGenerator): def onload(self): @@ -83,15 +94,22 @@ def before_insert(self): if not self.page_title: self.page_title = "My Page" if not self.route: - self.route = ( - f"pages/{camel_case_to_kebab_case(self.page_title, True)}-{frappe.generate_hash(length=4)}" - ) + if not self.name: + self.autoname() + self.route = f"pages/{self.name}" def on_update(self): + if self.has_value_changed("route"): + if ":" in self.route or "<" in self.route: + self.db_set("dynamic_route", 1) + else: + self.db_set("dynamic_route", 0) + if ( self.has_value_changed("dynamic_route") or self.has_value_changed("route") or self.has_value_changed("published") + or self.has_value_changed("disable_indexing") ): self.clear_route_cache() @@ -131,8 +149,9 @@ def add_comment(self, comment_type="Comment", text=None, comment_email=None, com ) @frappe.whitelist() - def publish(self, **kwargs): - frappe.form_dict.update(kwargs) + def publish(self, route_variables=None): + if route_variables: + frappe.form_dict.update(frappe.parse_json(route_variables or "{}")) self.published = 1 if self.draft_blocks: self.blocks = self.draft_blocks @@ -155,6 +174,7 @@ def unpublish(self, **kwargs): def get_context(self, context): # delete default favicon del context.favicon + context.disable_indexing = self.disable_indexing page_data = self.get_page_data() if page_data.get("title"): context.title = page_data.get("page_title") @@ -169,10 +189,12 @@ def get_context(self, context): blocks = self.draft_blocks content, style, fonts = get_block_html(blocks) + self.set_custom_font(context, fonts) context.fonts = fonts context.content = content context.style = render_template(style, page_data) context.editor_link = f"/{builder_path}/page/{self.name}" + context.page_name = self.name if self.dynamic_route and hasattr(frappe.local, "request"): context.base_url = frappe.utils.get_url(frappe.local.request.path or self.route) @@ -183,7 +205,6 @@ def get_context(self, context): context.update(page_data) self.set_meta_tags(context=context, page_data=page_data) self.set_favicon(context) - try: context["content"] = render_template(context.content, context) except TemplateSyntaxError: @@ -228,17 +249,13 @@ def set_style_and_script(self, context): context.setdefault("styles", []).append(builder_settings.style_public_url) @frappe.whitelist() - def get_page_data(self, args=None): - if args: - args = frappe.parse_json(args) - frappe.form_dict.update(args) + def get_page_data(self, route_variables=None): + if route_variables: + frappe.form_dict.update(frappe.parse_json(route_variables or "{}")) page_data = frappe._dict() if self.page_data_script: _locals = dict(data=frappe._dict()) - if is_safe_exec_enabled(): - safe_exec(self.page_data_script, None, _locals, script_filename=self.name) - else: - safer_exec(self.page_data_script, None, _locals, script_filename=self.name) + execute_script(self.page_data_script, _locals, self.name) page_data.update(_locals["data"]) return page_data @@ -250,6 +267,15 @@ def generate_page_preview_image(self, html=None): ) self.db_set("preview", public_path, commit=True, update_modified=False) + def set_custom_font(self, context, font_map): + user_fonts = frappe.get_all( + "User Font", fields=["font_name", "font_file"], filters={"name": ("in", list(font_map.keys()))} + ) + if user_fonts: + context.custom_fonts = user_fonts + for font in user_fonts: + del font_map[font.font_name] + def save_as_template(page_doc: BuilderPage): # move all assets to www/builder_assets/{page_name} @@ -354,7 +380,7 @@ def get_tag(block, soup, data_key=None): tag[key] = value if block.get("baseStyles", {}): - style_class = f"frappe-builder-{frappe.generate_hash(length=8)}" + style_class = f"fb-{frappe.generate_hash(length=8)}" base_styles = block.get("baseStyles", {}) mobile_styles = block.get("mobileStyles", {}) tablet_styles = block.get("tabletStyles", {}) @@ -378,7 +404,7 @@ def get_tag(block, soup, data_key=None): ) classes.insert(0, style_class) - tag.attrs["class"] = get_class(classes) + tag.attrs["class"] = " ".join(classes) innerContent = block.get("innerHTML") if innerContent: @@ -432,19 +458,6 @@ def get_style(style_obj): ) -def get_class(class_list): - return " ".join(class_list) - - -def camel_case_to_kebab_case(text, remove_spaces=False): - if not text: - return "" - text = re.sub(r"(? str: - # to load preview without publishing - frappe.form_dict.update(kwarg) - renderer = BuilderPageRenderer(path="") - renderer.docname = page - renderer.doctype = "Builder Page" - frappe.flags.show_preview = True - frappe.local.no_cache = 1 - renderer.init_context() - response = renderer.render() - page = frappe.get_cached_doc("Builder Page", page) - frappe.enqueue_doc( - page.doctype, - page.name, - "generate_page_preview_image", - html=str(response.data, "utf-8"), - queue="short", - ) - return response - - @redis_cache(ttl=60 * 60) def find_page_with_path(route): try: @@ -664,176 +608,15 @@ def get_web_pages_with_dynamic_routes() -> dict[str, str]: def resolve_path(path): - if find_page_with_path(path): - return path - elif evaluate_dynamic_routes( - [Rule(f"/{d.route}", endpoint=d.name) for d in get_web_pages_with_dynamic_routes()], - path, - ): - return path + try: + if find_page_with_path(path): + return path + elif evaluate_dynamic_routes( + [ColonRule(f"/{d.route}", endpoint=d.name) for d in get_web_pages_with_dynamic_routes()], + path, + ): + return path + except Exception: + pass return original_resolve_path(path) - - -def is_component_used(blocks, component_id): - blocks = frappe.parse_json(blocks) - if not isinstance(blocks, list): - blocks = [blocks] - - for block in blocks: - if not block: - continue - if block.get("extendedFromComponent") == component_id: - return True - elif block.get("children"): - return is_component_used(block.get("children"), component_id) - - return False - - -def copy_img_to_asset_folder(block, self): - if block.get("element") == "img": - src = block.get("attributes", {}).get("src") - site_url = frappe.utils.get_url() - - if src and (src.startswith(f"{site_url}/files") or src.startswith("/files")): - # find file doc - if src.startswith(f"{site_url}/files"): - src = src.split(f"{site_url}")[1] - # url decode - src = unquote(src) - print(f"src: {src}") - files = frappe.get_all("File", filters={"file_url": src}, fields=["name"]) - print(f"files: {files}") - if files: - _file = frappe.get_doc("File", files[0].name) - # copy physical file to new location - assets_folder_path = get_template_assets_folder_path(self) - shutil.copy(_file.get_full_path(), assets_folder_path) - block["attributes"]["src"] = f"/builder_assets/{self.name}/{src.split('/')[-1]}" - for child in block.get("children", []): - copy_img_to_asset_folder(child, self) - - -def get_builder_page_preview_paths(page_doc): - public_path, public_path = None, None - if page_doc.is_template: - local_path = os.path.join(get_template_assets_folder_path(page_doc), "preview.jpeg") - public_path = f"/builder_assets/{page_doc.name}/preview.jpeg" - else: - file_name = f"{page_doc.name}-preview.jpeg" - local_path = os.path.join(frappe.local.site_path, "public", "files", file_name) - random_hash = frappe.generate_hash(length=5) - public_path = f"/files/{file_name}?v={random_hash}" - return public_path, local_path - - -def get_template_assets_folder_path(page_doc): - path = os.path.join(frappe.get_app_path("builder"), "www", "builder_assets", page_doc.name) - if not os.path.exists(path): - os.makedirs(path) - return path - - -@frappe.whitelist() -def upload_builder_asset(): - from frappe.handler import upload_file - - image_file = upload_file() - if image_file.file_url.endswith((".png", ".jpeg", ".jpg")) and frappe.get_cached_value( - "Builder Settings", None, "auto_convert_images_to_webp" - ): - convert_to_webp(file_doc=image_file) - return image_file - - -@frappe.whitelist() -def convert_to_webp(image_url: str | None = None, file_doc: Document | None = None) -> str: - """BETA: Convert image to webp format""" - - CONVERTIBLE_IMAGE_EXTENSIONS = ["png", "jpeg", "jpg"] - - def can_convert_image(extn): - return extn.lower() in CONVERTIBLE_IMAGE_EXTENSIONS - - def get_extension(filename): - return filename.split(".")[-1].lower() - - def convert_and_save_image(image, path): - image.save(path, "WEBP") - return path - - def update_file_doc_with_webp(file_doc, image, extn): - webp_path = file_doc.get_full_path().replace(extn, "webp") - convert_and_save_image(image, webp_path) - delete_file(file_doc.get_full_path()) - file_doc.file_url = f"{file_doc.file_url.replace(extn, 'webp')}" - file_doc.save() - return file_doc.file_url - - def create_new_webp_file_doc(file_url, image, extn): - files = frappe.get_all("File", filters={"file_url": file_url}, fields=["name"], limit=1) - if files: - _file = frappe.get_doc("File", files[0].name) - webp_path = _file.get_full_path().replace(extn, "webp") - convert_and_save_image(image, webp_path) - new_file = frappe.copy_doc(_file) - new_file.file_name = f"{_file.file_name.replace(extn, 'webp')}" - new_file.file_url = f"{_file.file_url.replace(extn, 'webp')}" - new_file.save() - return new_file.file_url - return file_url - - def handle_image_from_url(image_url): - image_url = unquote(image_url) - response = requests.get(image_url) - image = Image.open(BytesIO(response.content)) - filename = image_url.split("/")[-1] - extn = get_extension(filename) - if can_convert_image(extn): - _file = frappe.get_doc( - { - "doctype": "File", - "file_name": f"{filename.replace(extn, 'webp')}", - "file_url": f"/files/{filename.replace(extn, 'webp')}", - } - ) - webp_path = _file.get_full_path() - convert_and_save_image(image, webp_path) - _file.save() - return _file.file_url - return image_url - - if not image_url and not file_doc: - return "" - - if file_doc: - if file_doc.file_url.startswith("/files"): - image, filename, extn = get_local_image(file_doc.file_url) - if can_convert_image(extn): - return update_file_doc_with_webp(file_doc, image, extn) - return file_doc.file_url - - if image_url.startswith("/files"): - image, filename, extn = get_local_image(image_url) - if can_convert_image(extn): - return create_new_webp_file_doc(image_url, image, extn) - return image_url - - if image_url.startswith("/builder_assets"): - image_path = os.path.abspath(frappe.get_app_path("builder", "www", image_url.lstrip("/"))) - image_path = image_path.replace("_", "-") - image_path = image_path.replace("/builder-assets", "/builder_assets") - - image = Image.open(image_path) - extn = get_extension(image_path) - if can_convert_image(extn): - webp_path = image_path.replace(extn, "webp") - convert_and_save_image(image, webp_path) - return image_url.replace(extn, "webp") - return image_url - - if image_url.startswith("http"): - return handle_image_from_url(image_url) - - return image_url diff --git a/builder/builder/doctype/builder_page/patches/create_upload_folder_for_builder.py b/builder/builder/doctype/builder_page/patches/create_upload_folder_for_builder.py index adbeaa7d..c68c770f 100644 --- a/builder/builder/doctype/builder_page/patches/create_upload_folder_for_builder.py +++ b/builder/builder/doctype/builder_page/patches/create_upload_folder_for_builder.py @@ -1,7 +1,8 @@ - import frappe from frappe.core.api.file import create_new_folder + def execute(): """create upload folder for builder""" - create_new_folder("Builder Uploads", "Home") \ No newline at end of file + create_new_folder("Builder Uploads", "Home") + create_new_folder("Fonts", "Home/Builder Uploads") diff --git a/builder/builder/doctype/builder_page/test_builder_page.py b/builder/builder/doctype/builder_page/test_builder_page.py index 451254d5..e3cbe4a7 100644 --- a/builder/builder/doctype/builder_page/test_builder_page.py +++ b/builder/builder/doctype/builder_page/test_builder_page.py @@ -52,7 +52,7 @@ def test_publish_unpublish(self): from frappe.utils import get_html_for_route content = get_html_for_route("/test-page") - self.assertTrue("The page you are looking for has gone missing" in content) + self.assertTrue("window.is_404 = true;" in content) self.page.publish() content = get_response_content("/test-page") diff --git a/builder/builder/doctype/builder_settings/builder_settings.json b/builder/builder/doctype/builder_settings/builder_settings.json index 242ec152..e515ef35 100644 --- a/builder/builder/doctype/builder_settings/builder_settings.json +++ b/builder/builder/doctype/builder_settings/builder_settings.json @@ -57,7 +57,7 @@ }, { "default": "1", - "description": "Beta: All the images uploaded to Canvas will be auto converted to WebP for better page performance.", + "description": "All the images uploaded to Canvas will be auto converted to WebP for better page performance.", "fieldname": "auto_convert_images_to_webp", "fieldtype": "Check", "label": "Auto convert images to WebP" diff --git a/builder/builder/doctype/user_font/__init__.py b/builder/builder/doctype/user_font/__init__.py new file mode 100644 index 00000000..e69de29b diff --git a/builder/builder/doctype/user_font/test_user_font.py b/builder/builder/doctype/user_font/test_user_font.py new file mode 100644 index 00000000..fdf4ca1a --- /dev/null +++ b/builder/builder/doctype/user_font/test_user_font.py @@ -0,0 +1,9 @@ +# Copyright (c) 2024, Frappe Technologies Pvt Ltd and Contributors +# See license.txt + +# import frappe +from frappe.tests.utils import FrappeTestCase + + +class TestUserFont(FrappeTestCase): + pass diff --git a/builder/builder/doctype/user_font/user_font.js b/builder/builder/doctype/user_font/user_font.js new file mode 100644 index 00000000..0b4d4d27 --- /dev/null +++ b/builder/builder/doctype/user_font/user_font.js @@ -0,0 +1,8 @@ +// Copyright (c) 2024, Frappe Technologies Pvt Ltd and contributors +// For license information, please see license.txt + +// frappe.ui.form.on("User Font", { +// refresh(frm) { + +// }, +// }); diff --git a/builder/builder/doctype/user_font/user_font.json b/builder/builder/doctype/user_font/user_font.json new file mode 100644 index 00000000..815c0bdf --- /dev/null +++ b/builder/builder/doctype/user_font/user_font.json @@ -0,0 +1,62 @@ +{ + "actions": [], + "allow_rename": 1, + "autoname": "field:font_name", + "creation": "2024-09-23 11:43:02.981496", + "doctype": "DocType", + "engine": "InnoDB", + "field_order": [ + "font_name", + "font_file" + ], + "fields": [ + { + "fieldname": "font_name", + "fieldtype": "Data", + "label": "Font Name", + "unique": 1 + }, + { + "fieldname": "font_file", + "fieldtype": "Attach", + "label": "Font File" + } + ], + "index_web_pages_for_search": 1, + "links": [], + "modified": "2024-09-26 11:24:22.897525", + "modified_by": "Administrator", + "module": "Builder", + "name": "User Font", + "naming_rule": "By fieldname", + "owner": "Administrator", + "permissions": [ + { + "create": 1, + "delete": 1, + "email": 1, + "export": 1, + "print": 1, + "read": 1, + "report": 1, + "role": "System Manager", + "share": 1, + "write": 1 + }, + { + "create": 1, + "delete": 1, + "email": 1, + "export": 1, + "print": 1, + "read": 1, + "report": 1, + "role": "Website Manager", + "share": 1, + "write": 1 + } + ], + "sort_field": "creation", + "sort_order": "DESC", + "states": [] +} \ No newline at end of file diff --git a/builder/builder/doctype/user_font/user_font.py b/builder/builder/doctype/user_font/user_font.py new file mode 100644 index 00000000..b0a84858 --- /dev/null +++ b/builder/builder/doctype/user_font/user_font.py @@ -0,0 +1,9 @@ +# Copyright (c) 2024, Frappe Technologies Pvt Ltd and contributors +# For license information, please see license.txt + +# import frappe +from frappe.model.document import Document + + +class UserFont(Document): + pass diff --git a/builder/builder/test_utils.py b/builder/builder/test_utils.py new file mode 100644 index 00000000..cd3822d7 --- /dev/null +++ b/builder/builder/test_utils.py @@ -0,0 +1,81 @@ +from unittest.mock import patch + +import frappe +from frappe.tests.utils import FrappeTestCase + +from builder.utils import ( + camel_case_to_kebab_case, + escape_single_quotes, + execute_script, + get_builder_page_preview_paths, + get_dummy_blocks, + get_template_assets_folder_path, + is_component_used, + remove_unsafe_fields, +) + + +class TestBuilderPage(FrappeTestCase): + def test_camel_case_to_kebab_case(self): + self.assertEqual(camel_case_to_kebab_case("backgroundColor"), "background-color") + self.assertEqual(camel_case_to_kebab_case("Color"), "color") + self.assertEqual(camel_case_to_kebab_case("color"), "color") + self.assertEqual(camel_case_to_kebab_case("NewPage"), "new-page") + self.assertEqual(camel_case_to_kebab_case("new page", remove_spaces=True), "newpage") + + def test_escape_single_quotes(self): + self.assertEqual(escape_single_quotes("Hello 'World'"), "Hello \\'World\\'") + self.assertEqual(escape_single_quotes("Hello World"), "Hello World") + + def test_is_component_used(self): + dummy_blocks = get_dummy_blocks() + self.assertTrue(is_component_used(dummy_blocks, "component-1")) + self.assertTrue(is_component_used(dummy_blocks, "component-2")) + self.assertFalse(is_component_used(dummy_blocks, "component-3")) + + def test_get_builder_page_preview_paths(self): + page_doc = frappe._dict( + { + "name": "test-page", + "is_template": False, + } + ) + public_path, local_path = get_builder_page_preview_paths(page_doc) + self.assertRegex(public_path, r"/files/test-page-preview.jpeg\?v=\w{5}") + self.assertEqual(local_path, f"{frappe.local.site_path}/public/files/test-page-preview.jpeg") + + page_doc.is_template = True + public_path, local_path = get_builder_page_preview_paths(page_doc) + self.assertEqual(public_path, "/builder_assets/test-page/preview.jpeg") + self.assertEqual( + local_path, f"{frappe.get_app_path('builder')}/www/builder_assets/test-page/preview.jpeg" + ) + + def test_get_template_assets_folder_path(self): + page_doc = frappe._dict({"name": "mypage"}) + path = get_template_assets_folder_path(page_doc) + self.assertEqual(path, f"{frappe.get_app_path('builder')}/www/builder_assets/mypage") + + @patch("builder.utils.is_safe_exec_enabled", return_value=False) + @patch("frappe.utils.safe_exec.is_safe_exec_enabled", return_value=False) + def test_execute_script_with_enabled_server_script(self, *args): + script = "data.test = frappe.get_doc('User', 'Administrator').email" + _locals = dict(data=frappe._dict()) + execute_script(script, _locals, "test.py") + self.assertEqual(_locals["data"]["test"], "admin@example.com") + + @patch("builder.utils.is_safe_exec_enabled", return_value=True) + @patch("frappe.utils.safe_exec.is_safe_exec_enabled", return_value=True) + def test_execute_script_with_disabled_server_script(self, *args): + script = "data.test = frappe.get_doc('User', 'Administrator').email" + _locals = dict(data=frappe._dict()) + execute_script(script, _locals, "test.py") + self.assertEqual(_locals["data"]["test"], "admin@example.com") + + script = "data.users = frappe.db.get_all('User')" + execute_script(script, _locals, "test.py") + self.assertTrue(_locals["data"]["users"]) + + script = "data.users = frappe.db.get_all('User')" + execute_script(script, _locals, "test.py") + self.assertTrue(_locals["data"]["users"]) diff --git a/builder/install.py b/builder/install.py index 8333b112..203fc85a 100644 --- a/builder/install.py +++ b/builder/install.py @@ -6,6 +6,7 @@ def after_install(): create_new_folder("Builder Uploads", "Home") + create_new_folder("Fonts", "Home/Builder Uploads") sync_page_templates() sync_block_templates() diff --git a/builder/patches.txt b/builder/patches.txt index e0247868..20dcd179 100644 --- a/builder/patches.txt +++ b/builder/patches.txt @@ -1,5 +1,5 @@ [pre_model_sync] -builder.builder.doctype.builder_page.patches.create_upload_folder_for_builder # +builder.builder.doctype.builder_page.patches.create_upload_folder_for_builder # 25-09-2024 builder.builder.patches.rename_web_page_beta_to_builder_page builder.builder.patches.rename_web_page_component_to_builder_component @@ -8,3 +8,4 @@ builder.builder.doctype.builder_component.patches.set_component_id builder.builder.doctype.builder_page.patches.properly_extend_blocks_from_component builder.builder.doctype.builder_page.patches.attach_client_script_to_builder_page builder.builder.doctype.builder_page.patches.enable_auto_convert_to_webp_by_default +builder.builder.doctype.builder_client_script.patches.trigger_asset_compression diff --git a/builder/public/images/desk.png b/builder/public/images/desk.png new file mode 100644 index 00000000..f4a09599 Binary files /dev/null and b/builder/public/images/desk.png differ diff --git a/builder/public/js/identify.js b/builder/public/js/identify.js new file mode 100644 index 00000000..c5f57204 --- /dev/null +++ b/builder/public/js/identify.js @@ -0,0 +1,8 @@ +/** + * FingerprintJS v3.4.2 - Copyright (c) FingerprintJS, Inc, 2023 (https://fingerprint.com) + * Licensed under the MIT (http://www.opensource.org/licenses/mit-license.php) license. + * + * This software contains code from open-source projects: + * MurmurHash3 by Karan Lyons (https://github.com/karanlyons/murmurHash3.js) + */ +var e=function(){return e=Object.assign||function(e){for(var n,t=1,r=arguments.length;t