-
Notifications
You must be signed in to change notification settings - Fork 75
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: add sensitive env var clearing and e2e tests for session launch…
…er (#2701) ### TL;DR Added functionality to empty sensitive environment variables and updated form value synchronization. ### What changed? - Introduced `sensitivePatterns` array with regular expressions to identify sensitive environment variables. - Added `isSensitiveEnv` function to check if an environment variable is sensitive. - Implemented `emptySensitiveEnv` function to clear values of sensitive environment variables. - Updated `VFolderTableFormValues` interface to include `autoMountedFolderNames`. - Modified form value synchronization in `SessionLauncherPage` to omit specific fields and empty sensitive environment variables. - Unit test and E2E test for this change. ### How to test? 1. Navigate to the Session Launcher page. 2. Add environment variables with sensitive names (e.g., PASSWORD, SECRET_KEY). 3. Verify that sensitive environment variables are properly identified and their values are cleared when reloading browser. 4. Check if the URL updates correctly without including sensitive information. 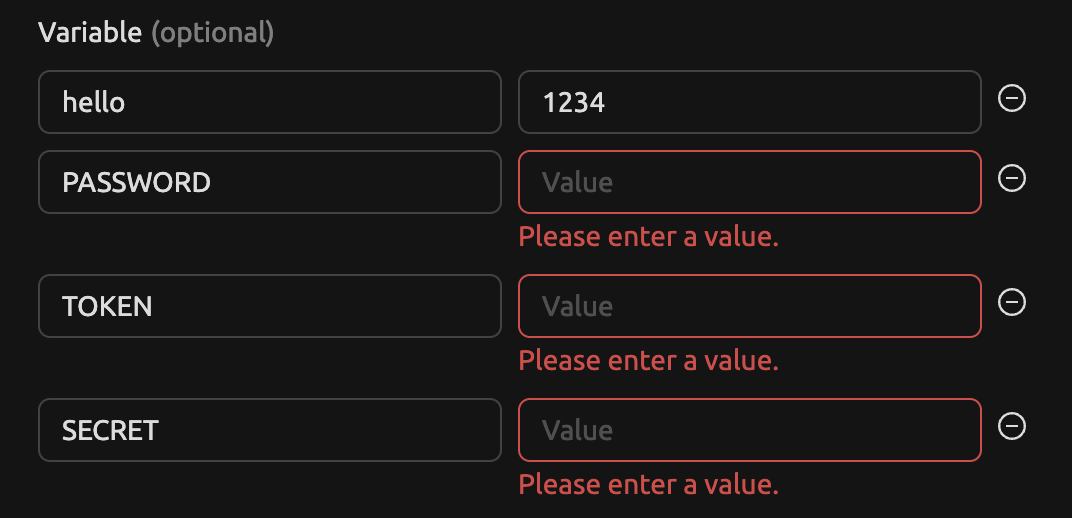 ### Why make this change? This change enhances security by preventing sensitive information from being exposed in URLs or unintended locations. It also improves the handling of environment variables, ensuring that sensitive data is properly managed throughout the application.
- Loading branch information
Showing
5 changed files
with
130 additions
and
14 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,35 @@ | ||
// EnvVarFormList.test.tsx | ||
import { sanitizeSensitiveEnv } from './EnvVarFormList'; | ||
|
||
describe('emptySensitiveEnv', () => { | ||
it('should empty the value of sensitive environment variables', () => { | ||
const envs = [ | ||
{ variable: 'SECRET_KEY', value: '12345' }, | ||
{ variable: 'API_KEY', value: 'abcdef' }, | ||
{ variable: 'NON_SENSITIVE', value: 'value' }, | ||
]; | ||
|
||
const result = sanitizeSensitiveEnv(envs); | ||
|
||
expect(result).toEqual([ | ||
{ variable: 'SECRET_KEY', value: '' }, | ||
{ variable: 'API_KEY', value: '' }, | ||
{ variable: 'NON_SENSITIVE', value: 'value' }, | ||
]); | ||
}); | ||
|
||
it('should not change non-sensitive environment variables', () => { | ||
const envs = [{ variable: 'NON_SENSITIVE', value: 'value' }]; | ||
const result = sanitizeSensitiveEnv(envs); | ||
|
||
expect(result).toEqual([{ variable: 'NON_SENSITIVE', value: 'value' }]); | ||
}); | ||
|
||
it('should handle an empty array', () => { | ||
const envs: any[] = []; | ||
|
||
const result = sanitizeSensitiveEnv(envs); | ||
|
||
expect(result).toEqual([]); | ||
}); | ||
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters